Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial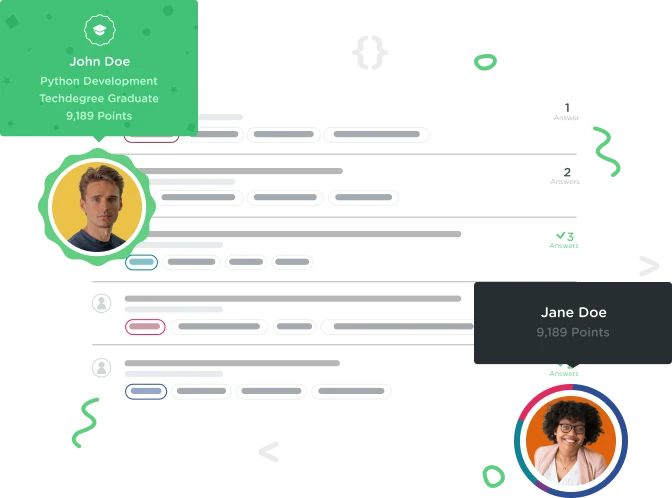
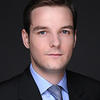
Bogdan Lalu
6,419 PointsUnable to complete the challenge at the end of the Python dictionaries course
Hi there, Can someone help me please spot what's missing in my code? I've tested it locally and to me it seems to work. The challenge asks to create a function that takes a string as an argument. It should return a dictionary with each word as key and the wordcount as a value. The challenge also suggests to lowercase the string for simplicity. When it fails it suggests to make sure I'm not splitting only based on spaces so I've used re. Here's my code below:
import re
def word_count(mystring):
result = {}
lower_mystring = mystring.lower()
initial_word_list = re.findall(r'\w+', lower_mystring)
unique_word_list = list(set(initial_word_list))
for word in unique_word_list:
result[word] = initial_word_list.count(word)
return result
Many thanks
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
import re
def word_count(mystring):
result = {}
lower_mystring = mystring.lower()
initial_word_list = re.findall(r'\w+', lower_mystring)
unique_word_list = list(set(initial_word_list))
for word in unique_word_list:
result[word] = initial_word_list.count(word)
return result
1 Answer
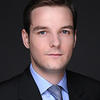
Bogdan Lalu
6,419 PointsThanks Dave for your code, it does work. I was so frustrated that mine didn't work that I did not see the big picture. My issue was somewhat different.
I solved mine after Kenneth suggested in a different post that I've misinterpreted the requirements of the challenge. This topic is closed now.
This is not about finding words in a sentence or paragraph but about splitting a string on spaces. Therefore a 'word' can contain punctuation. I wrongly assumed that it shouldn't, for the purpose of this challenge. So i've taken out the regex part and I just altered my code to what you see below. Not sure exactly why this code did not work in the first place though. I must have had a typo or something. The fact that it did not work made me overthink it and try to take out punctuation from words. Anyway the code below worked for me.
def word_count(mystring):
result = {}
lower_mystring = mystring.lower()
initial_word_list = lower_mystring.split()
unique_word_list = list(set(initial_word_list))
for word in unique_word_list:
result[word] = initial_word_list.count(word)
return result
Dave Laffan
4,604 PointsDave Laffan
4,604 PointsIf it helps, I used
Your code looks to be using words and commands I'm not yet familiar with so I'd be no help there sorry, but hopefully comparing my code might be of use.