Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial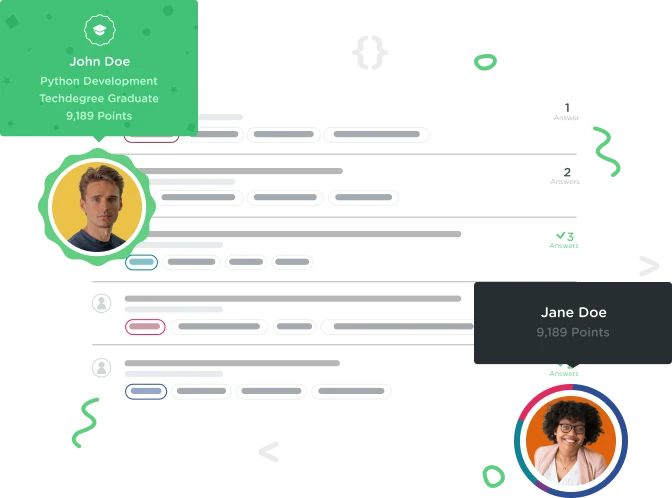
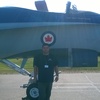
Arturo Espinoza
9,181 PointsUncaught TypeError: Cannot set property 'innerHTML' of null
Build a Quiz Challenge, Part 2 Solution
I am getting the following error on the console log: "Uncaught TypeError: Cannot set property 'innerHTML' of null."
Can someone please tell me what I am doing wrong. Thank you.
var questions = [
['How many states are in the US?', 50],
['How many continents are there?', 7],
['How many legs does an insect have?', 6]
];
var correctAnswers = 0;
var question;
var answer;
var response;
var correct = [];
var wrong = [];
function print(message) {
var outputDiv = document.getElementById('Output');
outputDiv.innerHTML = message;
}
function buildList(arr) {
var listHTML = '<ol>';
for (var i = 0; i < arr.length; i += 1) {
listHTML += '<li>' + arr[i] + '</li>';
}
listHTML += '</ol>';
return listHTML;
}
for (var i = 0; i < questions.length; i += 1){
question = questions[i][0];
answer = questions[i][1];
response = parseInt(prompt(question));
if (response === answer) {
correctAnswers += 1;
correct.push(question);
} else {
wrong.push(question);
}
}
html = "you got " + correct + " questions(s) right."
html += '<h2>You got theese questions wrong:</h2>';
html += buildList(correct);
html += '<h2>You got these questions correct:</h2>';
html += buildList(wrong);
print(html);
1 Answer

LaVaughn Haynes
12,397 PointsI can't see your HTML but it looks like you are having problems with this line
var outputDiv = document.getElementById('Output');
Make sure that there is an element in your HTML that has an ID of Output and that it's the same case. Output and output are different. Most likely you forgot to add the element to your HTML or it's the wrong case.
<!-- this HTML would work with your existing code -->
<div id="Output"></div>
<!-- you might have this HTML though -->
<div id="output"></div>
<!-- make sure your HTML and javaScript have the same case -->
I generally only use lowercase or camel case to avoid case issues because they can be tricky to spot
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Quiz</title>
</head>
<body>
<div id="Output"></div>
<script>
var questions = [
['How many states are in the US?', 50],
['How many continents are there?', 7],
['How many legs does an insect have?', 6]
];
var correctAnswers = 0;
var question;
var answer;
var response;
var correct = [];
var wrong = [];
function print(message) {
var outputDiv = document.getElementById('Output');
outputDiv.innerHTML = message;
}
function buildList(arr) {
var listHTML = '';
for (var i = 0; i < arr.length; i += 1) {
listHTML += '<li>' + arr[i] + '</li>';
}
listHTML += '';
return listHTML;
}
for (var i = 0; i < questions.length; i += 1){
question = questions[i][0];
answer = questions[i][1];
response = parseInt(prompt(question));
if (response === answer) {
correctAnswers += 1;
correct.push(question);
} else {
wrong.push(question);
}
}
html = "you got " + correct + " questions(s) right."
html += 'You got theese questions wrong:';
html += buildList(correct);
html += 'You got these questions correct:';
html += buildList(wrong);
print(html);
</script>
</body>
</html>
Arturo Espinoza
9,181 PointsArturo Espinoza
9,181 PointsOMG I can't believe I missed that. I checked everything like 50 times. Facepalm myself!! Thank you for your reply, you where right on the money.