Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial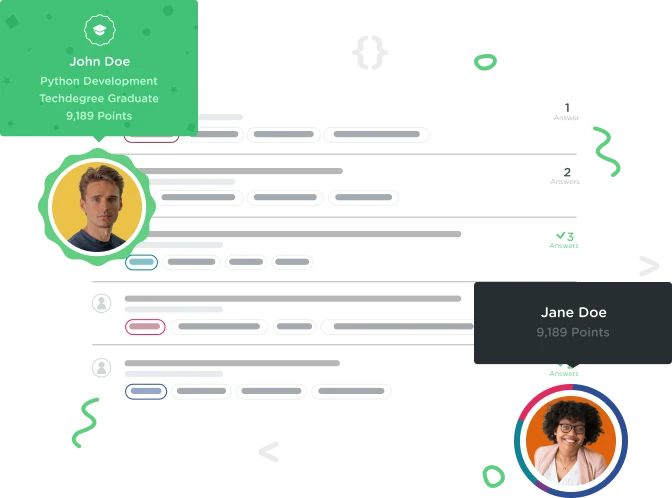

Neil Mosunic
3,436 PointsUndefined error: Mixing and Matching Arrays and Objects
In this lesson, we improved an old simple quiz script by changing the 2d array that held questions & answers to an array of objects.
This old simple quiz was also improved upon in a previous lesson, but the original, more simple version, of the quiz was used in this lesson to change the 2d array into an array of objects. I tried to also update the improved quizzes 2d array into an array of objects but am getting an undefined error for when the browser tries to display the correctly and incorrectly answered questions as a list. I know that in a 'for in' loop you cannot use dot notation. I tried changing all dot notation (for question and answer part of object) to bracket notation just in case, but that didn't help. Thanks for any help. Here is my code:
var questions = [
{
question: 'How many states are in the United States?',
answer: 50
},
{
question: 'How many continents are there?',
answer: 7
},
{
question: 'How many legs does an insect have?',
answer:6
}
];
var correctAnswers = 0;
var question;
var answer;
var response;
var correct = [];
var wrong = [];
var html;
function print (message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function buildList(arr) {
var listHTML = '<ol>';
for (var i = 0; i < arr.length; i += 1) {
listHTML += '<li>' + arr[i].question + '</li>';
}
listHTML += '</ol>';
return listHTML
} /* function to loop through arrays of correct & incorrectly answered questions and list the questions in an ordered list */
for (var i = 0; i < questions.length; i += 1) {
question = questions[i].question;
answer = questions[i].answer;
response = parseInt(prompt(question));
if (response === answer) {
correctAnswers += 1;
correct.push(question);
} else {
wrong.push(question);
}
}
html = "You got " + correctAnswers + " question(s) right.";
html += '<h2>You got these questions correct:</h2>';
html += buildList(correct);
html += '<h2>You got these questions wrong:</h2>';
html += buildList(wrong);
print(html);
2 Answers

Damien Watson
27,419 PointsHi Neil,
It's very close, the problem is that you are adding the question 'string' into the array, not the question 'object'.
You can do two approaches, either pass the full object into array:
correct.push(questions[i]);
or remove the .question
from inside the buildList
.
function buildList(arr) {
var listHTML = '<ol>';
for (var i = 0; i < arr.length; i += 1) {
listHTML += '<li>' + arr[i] + '</li>';
}
listHTML += '</ol>';
return listHTML
}

Dante Scavonni
433 PointsThank you Damien Watson !