Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial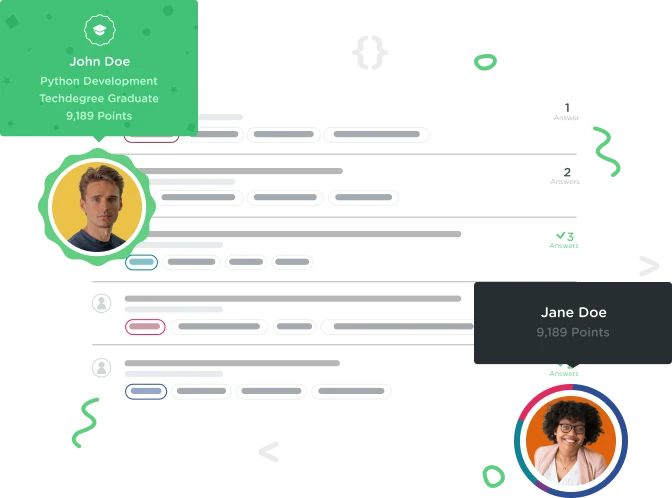
Hiromi De Young
2,294 PointsUnder the Ruby Boolean quiz, the question about a contains? method seems broken. Anyone else get it to work?
This code is 100% correct, yet the Ruby Boolean quiz refuses to accept it as a valid answer:
def contains?(name) @todo_items.include?(name) end
The system keeps saying "Bummer! The contains? method doesn't return a boolean value." Has anybody else been able to pass this question in order to move on?
class TodoList
attr_reader :name, :todo_items
def initialize(name)
@name = name
@todo_items = []
end
def add_item(name)
todo_items.push(TodoItem.new(name))
end
def contains?(name)
@todo_items.include?(name)
end
def find_index(name)
index = 0
found = false
todo_items.each do |item|
found = true if item.name == name
break if found
index += 1
end
if found
return index
else
return nil
end
end
end
t = TodoList.new('MyList')
t.todo_items << "test"
puts t.contains? "test"
1 Answer

Tim Knight
28,888 PointsHiromi,
What you have here is valid Ruby but I think they're looking for a little more from you. What if include?
didn't exist? How would you check to see if todo_items contained a value? You'd iterate over the array.
def contains?(name)
todo_items.each do |item|
return true if item.name == name
end
return false
end
Adrian Manteza
2,527 PointsAdrian Manteza
2,527 PointsHi Tim,
In the line
item.name == name ,is the .name a built in method? where is it defined?
Thanks
Tim Knight
28,888 PointsTim Knight
28,888 PointsAdrian, the ".name" is the "name" reader attribute defined right in the beginning. It is the name of the todo_item within the todo_items array that we're looping through and comparing it to the local value of "name" that we're passing into the function of "contains?".
Heather Stone
5,784 PointsHeather Stone
5,784 PointsHi Tim, I'm working on this challenge and wondering why it's not else return false, rather than just return false. Is the default state of the program to assume that the array doesn't have the item? To get existential on you, why is else used in some applications and not others? It seems like this would be a good place for it.
Tim Knight
28,888 PointsTim Knight
28,888 PointsHeather that's a really good question. The biggest thing to understand is that one you run a
return
in a method the method stops process anything else below it. So in this case we can just set a default return of false. If todo_items has an item that matchings thename
value we just return true which stops the method and returns. It's really a syntactical choice. You could just as well do a tertiary operator if you wanted to which is like if/else shorthandAgain though, it's really just a syntax choice. In my example we don't need else once it's true it'll stop... otherwise the else is inferred with the default
return
.