Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial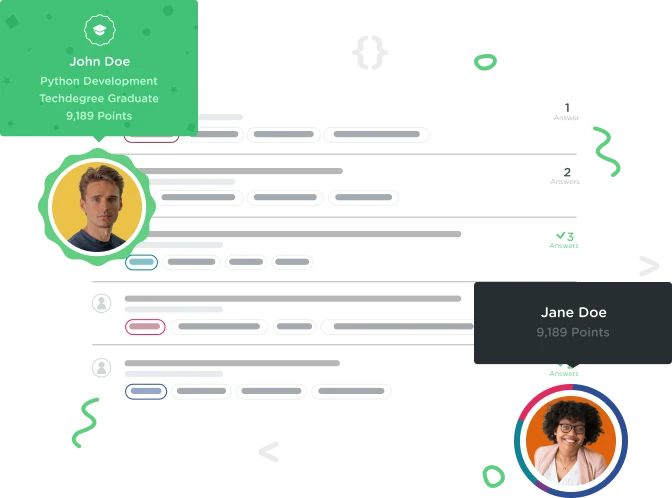

James Lowry
7,975 PointsUnreachable statement JSONObject currently = forecast.getJSONObject("currently");
I'm getting an error with this code:
JSONObject currently = forecast.getJSONObject("currently");
The code (JSONObject forecast = new JSONObject(jsonData);) shows no errors and was working properly on the other part of the project.
Android Studio tells me it is an unreachable statement. I checked the gradle app thinking maybe I'm missing something.
this is what I have for the dependencies... dependencies { compile fileTree(dir: 'libs', include: ['*.jar']) compile 'com.android.support:appcompat-v7:22.0.0' compile 'com.squareup.okhttp:okhttp:2.3.0' }
Maybe I accidentally deleted something or missed something.
Thanks in advance.

James Lowry
7,975 PointsThis is the main activity java file. Here is a link to the section. http://teamtreehouse.com/library/build-a-weather-app The error began in cleaning up the date and time.
import android.content.Context;
import android.net.ConnectivityManager;
import android.net.NetworkInfo;
import android.os.Bundle;
import android.support.v7.app.ActionBarActivity;
import android.util.Log;
import android.widget.Toast;
import com.squareup.okhttp.Call;
import com.squareup.okhttp.Callback;
import com.squareup.okhttp.OkHttpClient;
import com.squareup.okhttp.Request;
import com.squareup.okhttp.Response;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
public class MainActivity extends ActionBarActivity {
public static final String TAG = MainActivity.class.getSimpleName();
private CurrentWeather mCurrentWeather;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// begins API
String apiKey = "e38bf157d6b9de43ade1b64384348604";
Double longitude =-122.423;
Double latitude = 37.8267;
String forecastURL = "https://api.forecast.io/forecast//"
+apiKey+ "/"+latitude+ "," +longitude;
if(isNetworkAvailable()) {
OkHttpClient client = new OkHttpClient();
//begins request to get data
Request request = new Request.Builder()
.url(forecastURL)
.build();
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(Request request, IOException e) {
}
@Override
public void onResponse(Response response) throws IOException {
try {
String jsonData = response.body().string();
Log.v(TAG, jsonData);
// removed for streamlined codeResponse response = call.execute();
// must surround with a try catch block
if (response.isSuccessful()) {
mCurrentWeather = getCurrentDetails(jsonData);
}
else {
alertUserAboutError();
}
} catch (IOException e) {
Log.e(TAG, "Exception caught", e); // logs the exception to give a print out error
}
catch (JSONException e){
Log.e(TAG, "Exception caught", e);
}
}
});
}
else {
Toast.makeText(this, getString(R.string.network_unavailable), Toast.LENGTH_LONG).show();
}
// ends the get request
}
private CurrentWeather getCurrentDetails(String jsonData) throws JSONException {
JSONObject forecast = new JSONObject(jsonData);
String timeZone = forecast.getString("timezone");
Log.i(TAG, "From JSON" + timeZone);
return new CurrentWeather();
JSONObject currently = forecast.getJSONObject("currently");
CurrentWeather currentWeather = new CurrentWeather();
currentWeather.setHumidity(currently.getDouble("humidity"));
currentWeather.setTime(currently.getLong("time"));
currentWeather.setIcon(currently.getString("icon"));
currentWeather.setPrecipChance(currently.getDouble("precipProbability"));
currentWeather.setSummary(currently.getString("summary"));
currentWeather.setTemperature(currently.getDouble("temperature"));
currentWeather.setTimeZone(timeZone);
Log.d(TAG, currentWeather.getFormatedTime());
return currentWeather;
}
private boolean isNetworkAvailable() {
ConnectivityManager manager = (ConnectivityManager)
getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if (networkInfo != null && networkInfo.isConnected()){
isAvailable = true;
}
return isAvailable;
}
private void alertUserAboutError() {
AlertDialogFragment dialog = new AlertDialogFragment();
dialog.show(getFragmentManager(), "error_dialog");
}
}

James Lowry
7,975 PointsThis appears to be the only boolean method being called.
<p>
private boolean isNetworkAvailable() {
ConnectivityManager manager = (ConnectivityManager)
getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if (networkInfo != null && networkInfo.isConnected()){
isAvailable = true;
}
return isAvailable;
}
</p>
3 Answers
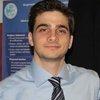
adamabdulghani
7,864 PointsHi James! Unreachable statement simply means that the statement and the following statements will never be reached. This is a logical error not a syntactical one. Your culprit is the return statement right before JSONObject currently = forecast.getJSONObject("currently"); Just remove the return statement and it will work. By removing this non conditional return statement the following statements will be reached.
Happy coding!

James Simshaw
28,738 PointsHello,
In your code:
private CurrentWeather getCurrentDetails(String jsonData) throws JSONException {
JSONObject forecast = new JSONObject(jsonData);
String timeZone = forecast.getString("timezone");
Log.i(TAG, "From JSON" + timeZone);
return new CurrentWeather();
JSONObject currently = forecast.getJSONObject("currently");
CurrentWeather currentWeather = new CurrentWeather();
currentWeather.setHumidity(currently.getDouble("humidity"));
currentWeather.setTime(currently.getLong("time"));
currentWeather.setIcon(currently.getString("icon"));
currentWeather.setPrecipChance(currently.getDouble("precipProbability"));
currentWeather.setSummary(currently.getString("summary"));
currentWeather.setTemperature(currently.getDouble("temperature"));
currentWeather.setTimeZone(timeZone);
Log.d(TAG, currentWeather.getFormatedTime());
return currentWeather;
}
You have "return new CurrentWeather();" after the Log.i() call in the middle of the function. Return returns the value and immediately exits the function, so anything after it is unreachable.

James Lowry
7,975 PointsThank you! I completely missed that I started after the return statement.

Eran Mani
3,751 PointsHello,
For some reason, i have a problem with my second json obejct - which is currently..i ran some tests and Log and saw that whenever the debugger reached the line of the currently json object, it stops completly. everything before it works great, such as time zone, but after that, nothing works. and i dont understand why. i followed ben examples, but still it wont work...really confusing..i will paste my code here..hope for help soon!
public class MainActivity extends AppCompatActivity {
CurrentWeather currentWeather;
@Bind(R.id.txtDegree) TextView degree;
@Bind(R.id.txtTime) TextView time;
@Bind(R.id.txtCity) TextView city;
@Bind(R.id.txtValueHumidiy) TextView humidity;
@Bind(R.id.txtSummery) TextView summery;
@Bind(R.id.txtPrecipValue) TextView precip;
@Bind(R.id.imageView2) ImageView icon;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//Binding the butter knife library to the activity
ButterKnife.bind(this);
String apiKey = "781c1591005925f1c96ad8b9a4147f74";
double latitude = 37.8267;
double longitude = -122.423;
String forecastUrl = "https://api.forecast.io/forecast/" + apiKey
+ "/" + latitude + "," + longitude;
if (isNetworkAvailable()) {
OkHttpClient client = new OkHttpClient();
final Request request = new Request.Builder()
.url(forecastUrl)
.build();
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(Request request, IOException e) {
}
@Override
public void onResponse(Response response) throws IOException {
try {
String jaston = response.body().string();
Log.v("Tag", jaston);
if (response.isSuccessful()) {
currentWeather = getCurrentDetails(jaston);
runOnUiThread(new Runnable() {
@Override
public void run() {
upadateDisplay();
}
});
} else {
alertUserAboutError();
}
} catch (IOException e) {
e.getMessage();
} catch (JSONException e) {
Log.i("Tag", "bla");
}
}
});
} else {
Toast.makeText(this, "network is not available!", Toast.LENGTH_SHORT).show();
}
Log.i("Tag", "Main UI is running!");
}
private void upadateDisplay() {
degree.setText(currentWeather.getTemperture() + "");
summery.setText(currentWeather.getSummery());
}
private CurrentWeather getCurrentDetails(String jsonData) throws JSONException {
JSONObject foreCast = new JSONObject(jsonData);
//WORKS!
String timeZone = foreCast.getString("timezone");
Log.i("Tag", timeZone);
String longitude = foreCast.getString("longitude");
Log.i("Tag", longitude);
JSONObject currently = foreCast.getJSONObject("currently");
CurrentWeather currentWeather = new CurrentWeather();
//NOT WORK!
double humidity = currently.getDouble("humdity");
Log.i("Tag", humidity + "");
String summery = currently.getString("summary");
Log.i("Tag", summery);
currentWeather.setHumidity(currently.getDouble("humdity"));
currentWeather.setTime(currently.getLong("time"));
currentWeather.setTemperture(currently.getDouble("temperature"));
currentWeather.setIcon(currently.getString("icon"));
currentWeather.setPrecipChance(currently.getDouble("precipProbability"));
currentWeather.setSummery(currently.getString("summary"));
currentWeather.setTimeZone(currently.getString(timeZone));
Log.i("Tag", currentWeather.getSummery());
return currentWeather;
}
private boolean isNetworkAvailable() {
ConnectivityManager manager = (ConnectivityManager) getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if (networkInfo != null && networkInfo.isConnected()) {
isAvailable = true;
}
return isAvailable;
}
private void alertUserAboutError() {
AlertDialogFragment dialogFragment = new AlertDialogFragment();
dialogFragment.show(getFragmentManager(), "error_dialog");
}
}
James Simshaw
28,738 PointsJames Simshaw
28,738 PointsCould you post your Java files? From my experience this tends to happen when you create a conditional that is always true or always false. For example: