Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial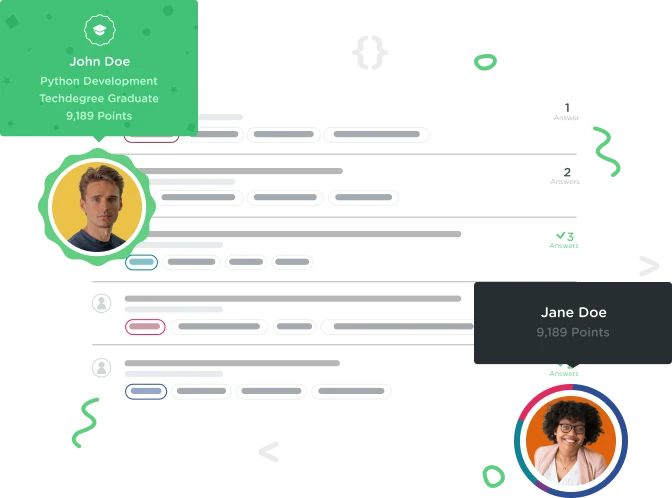

sahil shrestha
3,799 PointsUse a for or while loop to iterate through the values in the temperatures array from the first item -- 100 -- to the las
Use a for or while loop to iterate through the values in the temperatures array from the first item -- 100 -- to the last -- 10. Inside the loop, log the current array value to the console.
var temperatures = [100,90,99,80,70,65,30,10];
for ( i = 0 ; i < temperatures.length ; i += 1 ) {
console.log(temperatures);
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>

KRIS NIKOLAISEN
54,972 PointsOn the other hand if you use the index i that you created...
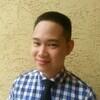
Tom Nguyen
33,502 PointsWhat it looks like to use the index:
console.log(temperatures[i]);
5 Answers
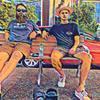
Frederick Bogdanoff
15,339 PointsYou have to use the variable you created inside of your for loop in order to loop through the array and log each array element individually.
for ( i = 0 ; i < temperatures.length ; i += 1 ) {
console.log(temperatures);
}
//The variable i starts with 0 as its value. It then adds 1 each time it loops.
//You can access array elements by using bracket notation.
//Since computers count from zero. You need to do this in order to grab the first element in the array.
temperatures[0]
//this will give you the first element, which is 100
//the interesting thing is that you've been storing numbers starting from 0 through to the length of the array inside of the for loop i variable.
for ( i = 0; i < temperatures.length; i += 1 ) {
console.log(temperatures[i]);
}
//Now you will be printing the right element for each iteration instead of printing the entire array through each iteration

kim corson
Full Stack JavaScript Techdegree Student 1,783 PointsThank you for helping with figuring out this problem. I am feeling absolutely stupid for this particular topic, I understand this may be a foolish request, but could someone break down for me a little bit more about how this code, 'knows' what the numbers in the array are. And additionally, why does the array iterator sometimes start at 0 and sometimes at 1?
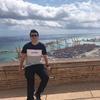
Tadjiev Codes
9,626 PointsHi,
Mr. Frederick, Hope all is going well... So it's not important to keep the keyword var before declaring the code i = 0; ? As I thought var i = 0; is like kinda mandatory.
Thanks in advance.

Adriana Cabrera
14,618 PointsHi i just want to add that treehouse will send u an error message if u don't use let to declare the variable i, but for sure this is the right way to do for ( let i = 0; i < temperatures.length; i += 1 ) { console.log(temperatures[i]); }

Sterling Gordon
5,376 PointsThanks for the explanation provided with notes, still trying to get a hold on how loops operate but this helps.

Mike Siwik
Full Stack JavaScript Techdegree Student 8,483 Points( i = 0; i < temperatures.length; i += 1 ) I didn't test this, but if anyone reads this, my code below passes.
const temperatures = {100, 90, 99, 80, 70, 65, 30, 10];
let items = '';
for (let i = 0; i < temperatures.length; i++) {
console.log(temperatures[i]);
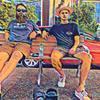
Frederick Bogdanoff
15,339 PointsYou shouldn't feel stupid dude, its the computers that are stupid! When you're programming you're basically giving instructions to a stupid machine. And there's no such thing as a foolish question dude :) I've been hung up by things, and still get hung up. It doesn't seem like it ever stops..
Where do I start? I guess we can start with how Arrays work. Arrays are a type of variable that can contain multiple elements under a single name, and you can access these elements by referring to an index number using bracket notation -> [] like in your temperatures array. The index of an array will always start at 0.
The for loop however can start from whatever index value you want. The for loop can be broken into 3 statements via: for (statement 1, statement 2, statement 3). statement 1 is your variable declaration, this is where you declare the start of your for loop iteration(var i = 0). Statement 2 is where the for loop checks the logic or condition of the for loop, for instance "while i is less then the length of temperatures"(i < temperatures.length). Statement 3 is executed every time the code block of your for loop is executed(temperatures += 1).
Because the value of the variable i is a number, and you can access elements of your array using bracket notation and a number via: temperature[3]. You can put the temperatures array inside of your for loop code block, and use i as your parameter for bracket notation on temperatures via temperature[i]. each time the code block runs, temperatures will return the element that is equal to the variable i in its index.
I hope I've explained this well enough. Being able to explain concepts is just as important as understanding them lol.
further reading: https://www.w3schools.com/js/js_arrays.asp and https://www.w3schools.com/js/js_loop_for.asp
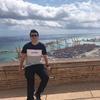
Tadjiev Codes
9,626 PointsAnd this explanation is great))))
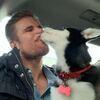
Skyler Harris
7,670 PointsThis right here ^ This explanation caught me up in 2 minutes, after feeling totally lost. Good on you, brothah!
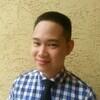
Tom Nguyen
33,502 PointsSolution:
const temperatures = [100, 90, 99, 80, 70, 65, 30, 10];
for ( var i = 0; i < temperatures.length; i ++ ) {
console.log(temperatures[i]);
}
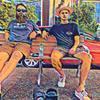
Frederick Bogdanoff
15,339 PointsHey Mukhammadkhon. Your second question goes a little bit further into the weird parts of javascript. And has to do with hoisting and variable scope.
I believe the best practice in most situations would be to include the var keyword in your for loop:
for (var i = 0; i < 5; i++)
using var inside of the for loop will reduce its scope and make room for less errors "Example being if you have another variable named i outside of the for loop"
But if you for some reason need to use the variable outside of the for loop you would have to declare it outside such as:
var i;
for (i = 0; i < 5; i++)
Apologies if my first answer confused you, I copied off of the example code in question.
:) hope your doing well! And glad I can help!
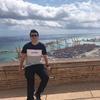
Tadjiev Codes
9,626 PointsAlright, Thanks a lot for the response. Appreciate it)))
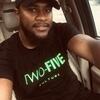
Andre Logan
9,897 Pointsfor ( let i = 0; i < temperatures.length; i += 1 ) { console.log(temperatures[i]); }
KRIS NIKOLAISEN
54,972 PointsKRIS NIKOLAISEN
54,972 PointsIf you run that through the javascript console you'll see
[100,90,99,80,70,65,30,10]
repeated 8 times.