Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial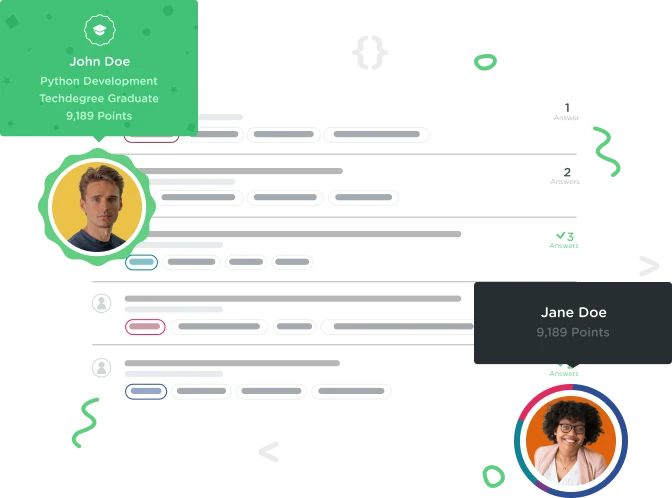
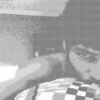
Bhupend Patil
25,918 PointsUsing a Model in the Controller. Code Challenge 3 of 3
Tried for more than 2hrs. Help me please :(
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
public class LandingActivity extends Activity {
public Button mThrustButton;
public TextView mTypeLabel;
public EditText mPassengersField;
public Spaceship mSpaceship;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_landing);
mThrustButton = (Button)findViewById(R.id.thrustButton);
mTypeLabel = (TextView)findViewById(R.id.typeTextView);
mPassengersField = (EditText)findViewById(R.id.passengersEditText);
// Add your code here!
mSpaceship = new Spaceship("FIREFLY");
mTypeLabel.setText(mSpaceship.getType());
}
}
public class Spaceship {
private String mType;
private int mNumPassengers = 0;
public String getType() {
return mType;
}
public void setType(String type) {
mType = type;
}
public int getNumPassengers() {
return mNumPassengers;
}
public void setNumPassengers(int numPassengers) {
mNumPassengers = numPassengers;
}
public Spaceship() {
mType = "SHUTTLE";
}
public Spaceship(String type) {
mType = type;
}
public Spaceship getNumPassenger(int numPassengers) {
return mSpaceship[numPassengers];
}
}
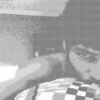
Bhupend Patil
25,918 PointsThankyou Sir it helped me :D
1 Answer

Milan Tailor
5,132 PointsLooks like I was overcomplicating it the files should be:
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
public class LandingActivity extends Activity {
public Button mThrustButton;
public TextView mTypeLabel;
public EditText mPassengersField;
public Spaceship mSpaceship;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_landing);
mThrustButton = (Button)findViewById(R.id.thrustButton);
mTypeLabel = (TextView)findViewById(R.id.typeTextView);
mPassengersField = (EditText)findViewById(R.id.passengersEditText);
// Add your code here!
//setup new spaceship
mSpaceship = new Spaceship("FIREFLY");
// Update TextView to use Spaceship's type
mTypeLabel.setText(mSpaceship.getType());
mPassengersField.setText("" + mSpaceship.getNumPassengers());
}
}
public class Spaceship {
private String mType;
private int mNumPassengers = 0;
public String getType() {
return mType;
}
public void setType(String type) {
mType = type;
}
public int getNumPassengers() {
return mNumPassengers;
}
public void setNumPassengers(int numPassengers) {
mNumPassengers = numPassengers;
}
public Spaceship() {
mType = "SHUTTLE";
}
public Spaceship(String type) {
mType = type;
}
}
So I think, the number of passengers is already set in the second file so we just need to get them in the first file, like mPassengersField.setText("" + mSpaceship.getNumPassengers());
The speech marks are just there to quickly convert an int to a string.
It passed, so I'm hoping it's right!
Milan Tailor
5,132 PointsMilan Tailor
5,132 PointsAt the stage as you with this one, I think I'm almost there, the only pop up error Im getting is with mPassengersField requiring a string but getting an int. Of course, as with all of this I could be completely wrong. You found the solution? How to convert from a string to int, brain work...