Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial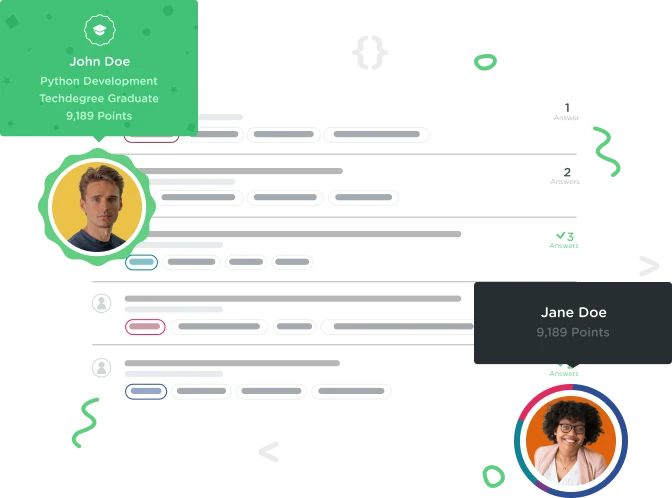

Edward Harvey
Courses Plus Student 6,899 PointsUsing Classes in Ruby
Hi, I am still learning so apologies about the stupid question, but here goes:-
I'm modifying the Bank Account program we learned in Ruby to allow it to take inputs using 'gets' to assign the account holder's name (& also a description & amount for transactions). I was wanting to do this using another Class to see if I can get two classes working together. I'm not sure if this is something that I shouldn't do - so I apologise if it is - but I am struggling to get the method to accept the input without running the puts method twice.
I would appreciate some advice on why it is doing this - or even better some way I should write the code to do what I want, so I can better understand how this kind of problem would be solved. I know my program is not very useful but am still just trying to figure out how Ruby works!
Many thanks in advance.
class BankAccount
attr_reader :name
def initialize(name)
@name = name
@transactions = []
add_transaction("Beginning Balance", 0)
end
def credit(description, amount)
add_transaction(description, amount)
end
def debit(description, amount)
add_transaction(description, -amount)
end
def add_transaction(description, amount)
@transactions.push(description: description, amount: amount)
end
def balance
balance = 0.0
@transactions.each do |transaction|
balance += transaction[:amount]
end
return balance
end
def print_register
puts "#{name}'s bank account"
@transactions.each do |transaction|
puts transaction[:description], transaction[:amount]
puts "\n"
end
puts "#{balance}"
end
end
class Admin
attr_reader :get_name
def initialize
@get_name = get_name
end
def get_name
puts "Please enter the Account Holders name: "
@get_name = gets.chomp
end
def get_description
puts "please a description: "
@get_description = gets.chomp
end
def get_amount
puts "please enter the amount: "
@get_amount = gets.to_i
end
end
admin = Admin.new
name = admin.get_name
bank_account = BankAccount.new(name)
bank_account.print_register
1 Answer

Edwin Herrera
13,106 Pointsthe problem is you defined your get_name
method along with an attr_reader
with the same name!
remember that attr_reader :get_name
is pretty much just a shortcut for:
def get_name
@get_name
end
this means that you're overwriting your 'attr_reader' with a different behaviour!
simply either rename your attr_reader to something like
attr_reader :name
or rename your method for getting the name input:
def get_name_from_input
....
end
although i'd recommend the former option!
Edward Harvey
Courses Plus Student 6,899 PointsEdward Harvey
Courses Plus Student 6,899 PointsI guess it's because I ran an initialize method? I'm still figuring out why that actually needs to be run. Could someone explain what the purpose of the initialize method is, I'm still confused by it.
Thanks!