Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial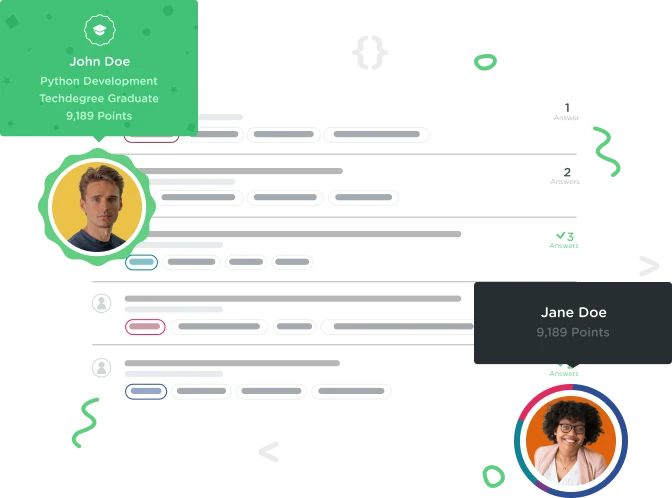

J V
3,414 PointsUsing For Loops with Arrays: Need calrification in regards to function printList(list) { }
/Code useful for program to run/
var ShoppingList = [
"Eggs",
"Tomatoes",
"Milk",
"Cake Mix",
"Onions",
"Pumkin",
"Stuffing"
];
function print(message)//message parameter
{
document.write(message);
}
I am having difficulty understanding the logic of how the function printList() operates in this example.
Here is the code:
function printList( list )
{
var listHTML = '<ol>'; /*line 3*/
for(i = 0; i < list.length; i++)
{
listHTML = listHTML + '<li>' + list[i] + '</li>'; /*line 7*/
}
listHTML = listHTML + '</ol>'; /*line 9*/
print(listHTML); /*line 10*/
}
printList(ShoppingList);
How does a programmer know how to write the code within the function above? It seems logical, but difficult to visualize when writing code. There are so many listHTML variables that are being placed inside one another.
I understand the logic of how the line 7 works with line 9.
From my understanding, var listHTML in line 3 is replaced by the listHTML in line 7. So far we have:
listHTML = '<ol>' + '<li>' + list[i] + '</li>'; /*line7*/
In regards to line 9, listHTML is replaced by line 7. So far we have:
listHTML = '<ol>' + '<li>' + list[i] + '</li>' + '</ol>';
And finally we print out line 9, hence the print statement on line 10.
I tried to write the code in a more logical way, but it does not work properly. I am not sure what may be the problem. I think line 3 may be the problem, but I do not see how it affects the output.
function printList( list )
{
var listHTML = ' '; /*line 3*/
for(i = 0; i < list.length; i++)
{
listHTML = '<ol>' + '<li>' + list[i] + '</li>';
}
//close the ol tag and print it to the page
listHTML = listHTML + '</ol>';
print(listHTML);
}
printList(ShoppingList);
The output is 1. Stuffing, which does not make sense.
2 Answers
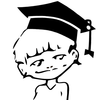
simhub
26,544 Pointshi jaafar, you have to concat the list tag "li" to the "ol" tag by iterating through the loop:
function printList( list )
{
var listHTML ='<ol>' ; /*line 3*/
for(i = 0; i < list.length; i++)
{
//here you concat a 'li' tag to 'ol' for every element in your array, by using [ += ]
listHTML += '<li>' + list[i] + '</li>';
}
//close the ol tag and print it to the page
listHTML = listHTML + '</ol>';
print(listHTML);
}

J V
3,414 PointsHi Dejan and simhub,
I appreciate your answers. Thank you for responding. I will have to go back and re-write the code for more practice and understanding.
deckey
14,630 Pointsdeckey
14,630 PointsHi, the problem with last code is that you print 'ol' inside the loop too! Basically getting list.length number of ordered lists, which is wrong. You should separate what is to be executed once inside printList() method and what should be executed repeatedly within the loop.
In this example, 'ol' should be called before the loop and closing '/ol' is called after the loop. Everything inside is repeated list.length number of times.
Usually when I do this kind of dynamic link creation, I write the pure html first, so, in your case: