Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial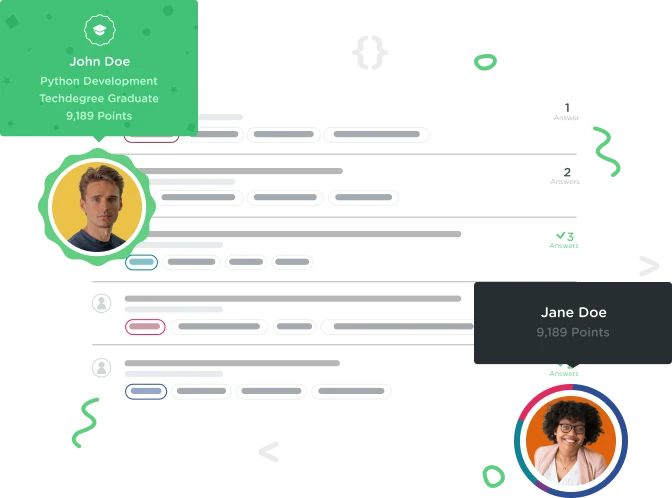

Hamilton Steele
8,717 PointsUsing nil vs false (specifically in find_index method)
So I just had a quick question about this part of Jason's code:
def find_index(name)
index = 0
found = false
todo_items.each do |todo_item|
if todo_item.name == name
found = true
end
if found
break
else
index += 1
end
end
if found
return index
else
return nil
end
end
specifically this part of the code...
if found
return index
else
return nil
end
why in this find_index method in the todo_list.rb do we return "nil" instead of just "false"?
what's the advantage of using nil if nil also evaluates to false anyways?
2 Answers
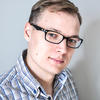
Damian Kosowski
19,746 PointsOne situation that pops into my mind is the difference between nil.nil? and false.nil? - the former evaluates to true the latter to false. So basically if we would use a control expression like - if variable.nil? do something and if not do something else - and we would get the variable from some earlier method, it would be important if that earlier method returns false or nil. I hope that makes sense. I would also love to hear if anyone has some other examples :)
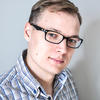
Damian Kosowski
19,746 PointsI would say that in this particular case we don't expect this method to return a true or false value. In Ruby methods that end with a question mark "?" by convention return a boolean. This method should just return the index and if it isn't found should return nothing.

Hamilton Steele
8,717 PointsYes, I understand that. But really, I'm just wondering, I guess functionally, what is the difference between nil and false? If nil evaluates as false anyways, why not use false? Is it simply just being more explicit for code readability or is there some other reason nil is best to use.
I guess what I'm asking is: I know that there is a difference between nil (or nothing) versus false, but is there a situation in ruby where using false instead of nil would produce something totally unexpected or is using nil just more explicit. I guess nil is the only instance of it's own class so it has its own methods that can work on it?
Thanks for the reply
Hamilton Steele
8,717 PointsHamilton Steele
8,717 PointsYes! That actually totally makes sense. Thanks!