Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial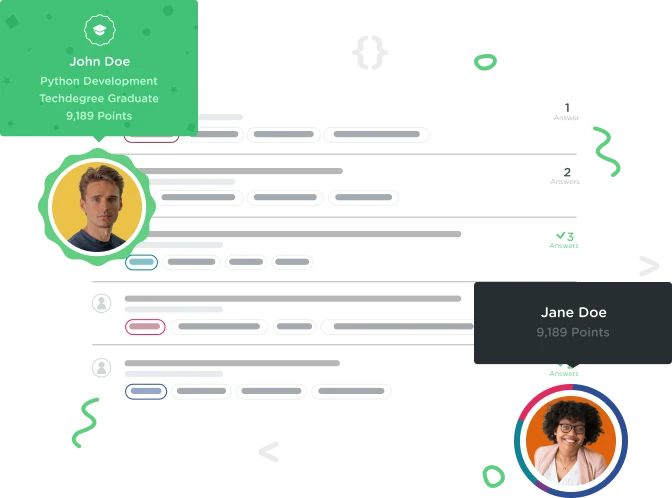
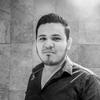
Ramiro Aguirre
Courses Plus Student 16,725 PointsUsing PDO within a Class
So i have this class
<?php
class Db
{
private $_connection;
private static $_instance; //The single instance
private $_host = 'localhost';
private $_port = 22737;
private $_username = 'user';
private $_password = 'password';
private $_database = 'databasename';
/*
Get an instance of the Database
@return Instance
*/
public static function getInstance()
{
if (!self::$_instance) { // If no instance then make one
self::$_instance = new self();
}
return self::$_instance;
}
// Constructor
private function __construct()
{
try {
$this->_connection = new \PDO("mysql:host=$this->_host;port=$this->_port;dbname=$this->_database", $this->_username, $this->_password);
/*** echo a message saying we have connected ***/
echo 'Connected to database';
} catch (PDOException $e) {
echo $e->getMessage();
}
}
// Magic method clone is empty to prevent duplication of connection
private function __clone()
{
}
// Get mysql pdo connection
public function getConnection()
{
return $this->_connection;
}
}
class Post {
public function __construct(){
$db = Db::getInstance();
$this->_dbh = $db->getConnection();
}
public function getPosts()
{
try {
/*** The SQL SELECT statement ***/
$sql = "CALL getStates";
foreach ($this->_dbh->query($sql) as $row) {
var_dump($row);
}
/*** close the database connection ***/
$this->_dbh = null;
} catch (PDOException $e) {
echo $e->getMessage();
}
}
}
?>
When i try to call it in the same file like this
<?php
$obj = new Post;
$obj->getPosts();
?>
i get nothing but the message Connected to database.
how can i get my data back?
i also tried this
<?php
$obj = new Post;
$obj->getPosts("CALL getStates");
?>
it gets me data but i dont think this is a good thing to do
2 Answers
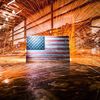
Andrew Shook
31,709 PointsSo it looks like you are using a stored procedure, are you sure the procedure is still available to the database? I know when I am developing I will need to wipe a DB and rebuild to make changes.
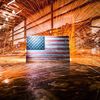
Andrew Shook
31,709 PointsNot sure why the stored procedure isn't working, but you could just change $sql to "SELECT * FROM posts".
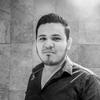
Ramiro Aguirre
Courses Plus Student 16,725 Pointsthe second try i did actually got me data back, but the thing is that i dont want to have to put the query as a parammetter i just want to have a simple instance and have that simple instance give me data without having to put the query between the parentesis.
Ramiro Aguirre
Courses Plus Student 16,725 PointsRamiro Aguirre
Courses Plus Student 16,725 Pointsyup ! it is still up there. what im trying to do is having a class for the Database connecion and another with the sql query i need so that when i use it in a file like index.php i don't write anything but the instance of my class there. for security reasons.