Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial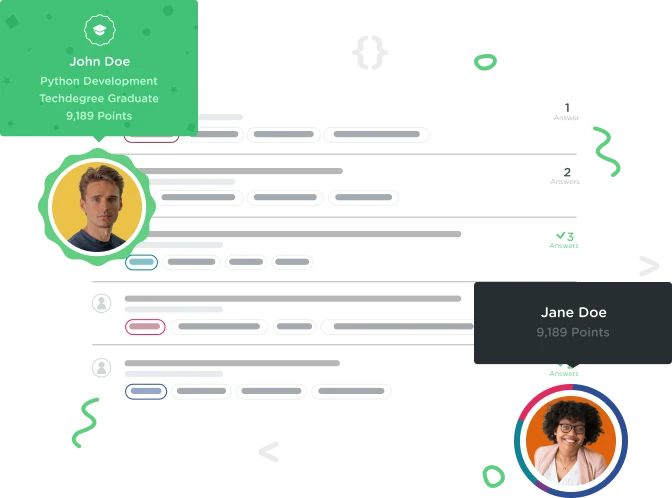
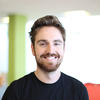
Kristian Woods
23,414 PointsUsing square brackets: Extend() vs Append()
Why when you use square brackets in append() - it creates a list inside a list?
array = [1,2,3]
array.append([4,5])
# results in: 1, 2, 3, [4, 5]]
However, when you use square brackets with extend() - it adds multiple values individually - i.e. NOT as a separate list:
array = [1,2,3]
array.extend([4,5])
# results in: 1, 2, 3, 4, 5]
so, to add a list within a list using extend, I'd use:
array = [1,2,3]
array.extend([[4,5]])
# results in: 1, 2, 3, [4, 5]]
This just seems confusing to me. I understand that append adds a single value, extend adds multiple values - Why does the behavior change between the two methods?
2 Answers

Greg Kaleka
39,021 PointsHi Kristian,
These two functions exist and behave differently because you might want to do one of two things:
- Add individual items to a list one at a time (
append
) - Add a list of items to a list all at once (
extend
)
Your last example works, but there's not really a good reason to use extend
like that, since you could just use append if you really wanted to add a list to the list. In fact, it's pretty rare that you would use your first example as well. It's just not that common to add an actual list to an existing list. You're more commonly going to want to add items. Python lists are super-flexible, but most of the time they'll just be a collection of the same thing.
Here's a realistic example: I've got a to-do web app, and I have an input on a website. If a user puts a single item into the input and hits submit, I run:
# todos: ["Go to the store", "Make dinner"]
# user inputs: "Make an appointment for next week"
# we run:
todos.append("Make an appointment for next week")
# todos: ["Go to the store", "Make dinner", "Make an appointment for next week"]
Now I add a feature where users can add multiple items at once using multiple input boxes. If they fill in multiple input boxes, the inputs come through as a list:
# todos: ["Go to the store", "Make dinner"]
# user inputs: ["Make an appointment", "Clean the kitchen"]
# we run:
todos.extend(["Make an appointment", "Clean the kitchen"])
# todos: ["Go to the store", "Make dinner", "Make an appointment", "Clean the kitchen"]
The short version is that for "one-dimensional" lists (ones that don't have other lists in them), append
is for adding one item, and extend
is for adding a list of items.
As you've discovered, there's more complexity than this, but 90% of the time this is how you'll use them.
Hope that helps!
Cheers
-Greg
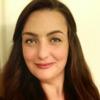
Jennifer Nordell
Treehouse TeacherHi there! The extends
function exists specifically so that it can add every item of an iterable to an already existing list. The append
item adds one object to a list. In your example, you append [4,5]
. That list is considered one object and in and of itself. The extend
breaks apart the iterable and adds each item separately. In your final example, you give array.extend([[4,5]])
. The argument there is a list, that contains exactly one item which is itself a list.
Take a look at this Python documentation. At the very top are the specs for the append and extend methods. As you'll see the append adds an object, while the extends takes an iterable and breaks it apart to put each item in the iterable onto the list you've designated.
I wrote this little example, which might help you see it better when you try it with a string.
greeting = []
greeting2 = []
greeting.append("Hi")
greeting.append("Kristian")
greeting2.extend("Hi")
greeting2.extend("Kristian")
print(greeting)
print(greeting2)
Hope this helps!