Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial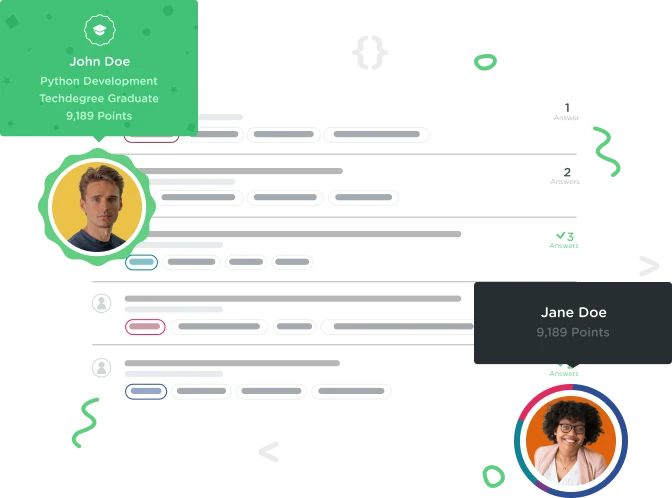

Antony Gabagul
9,351 PointsUsing until loop in ruby problem
Hey guys :) ! So I'm practicing my ruby and I'm trying to make function which sums all of the digits in a number until the sum is one digit number. Etc: 123 -> 1+2+3 = 6 99 -> 9+9 = 18 -> 1+8 =9 942 -> 9 + 4 + 2 = 15 -> 1+5 = 6 ... I'm trying to do this all using until loop but I always get first summation, my code doesn't keep to sum until i reaches one digit number. CODE:
n = gets.chomp.to_i
def digital_root(n)
#Takes n and splits its numbers into array, then it counts array
until n.to_s.chars.map(&:to_i).count == 1
#Sums ALL the values in the previously created array
n.to_s.chars.map(&:to_i).inject(0, :+)
return n
end
end
Thanks for any help :)
2 Answers

Ken jones
5,394 PointsHere's a solution i wrote that doesn't use the until loop...
def digital_root(n)
number = n
sum = 0
#splits our string into seperate charcters then turns them back into numbers
seperated_array = number.split(//)
seperated_array.map! { |n| n.to_i }
#loop to perform our maths
seperated_array.each do |number|
sum += number
end
return sum
end
print "Enter a number: "
n = gets.chomp.to_s
puts digital_root(n)
Ill try and get my head around using the until loop to do the same thing and see if i can post a solution!

Ken jones
5,394 PointsHeres the same thing but using the until loop.
def digital_root(n)
number = n
sum = 0
#splits our string into seperate charcters then turns them back into numbers
seperated_array = number.split(//)
seperated_array.map! { |n| n.to_i }
#loop to perform our maths
i = seperated_array.size
until i == 0
seperated_array.each do |number|
sum += number
i -= 1
end
end
return sum
end
print "Enter a number: "
n = gets.chomp.to_s
puts digital_root(n)