Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial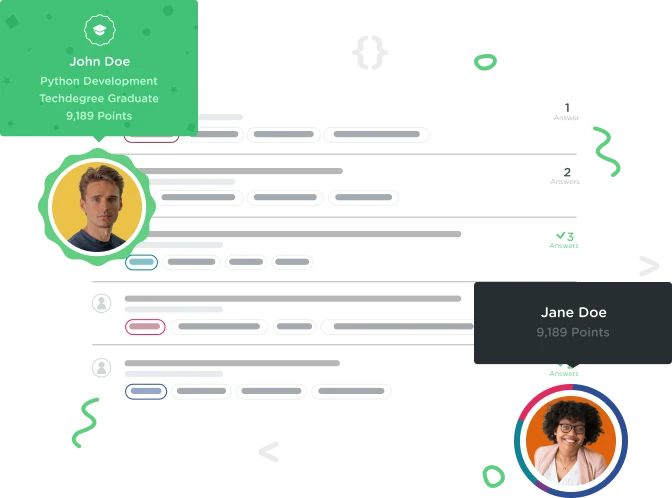
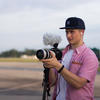
Christopher Johnson
12,829 PointsValidation check: else statement for non-existing record.
If I take out this line of code:
else {
print("Record not found.");
}
from:
var message = '';
var student;
var search;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function reportStudentReport (student) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
search = prompt("Enter name of student [Rob] or 'quit' to exit.");
//validation check
if (search === null || search.toLowerCase() === 'quit') {
break;
}
for (var i = 0; i < students.length; i++) {
student = students[i];
if (student.name.toLowerCase() === search.toLowerCase()) {
message = reportStudentReport(student);
print(message);
} else {
print("Record not found.");
}
}
}
Everything works as is; however, as simple as it should be, I can't get it to still check for student === search with an else statement below. I've checked for closing brackets and ;, which I think are all there.
1 Answer

ALFRED MOHENU
1,499 PointsYou will have to break out of the if statement after printing your message else your else statement will print a new message when you type quit. If you don't break out of your if statement, the complier goes on to read the else statement that is why it reads "record not found" regardless of what you type into the prompt.
var message = '';
var student;
var search;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function reportStudentReport (student) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
search = prompt("Enter name of student [Rob] or 'quit' to exit.");
//validation check
if (search === null || search.toLowerCase() === 'quit') {
break;
}
for (var i = 0; i < students.length; i++) {
student = students[i];
if (student.name.toLowerCase() === search.toLowerCase()) {
message = reportStudentReport(student);
print(message);
break;
}
else {
print("Record not found.");
}
}
}