Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial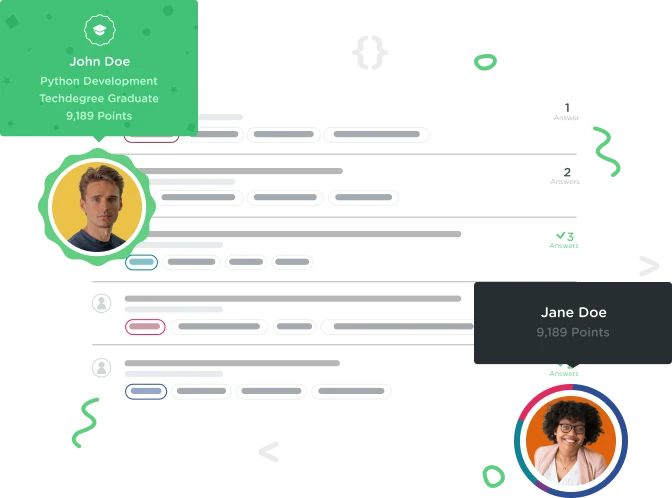
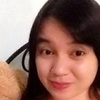
vanessa
3,455 Pointswas trying to use IndexOf but can't.. any comment on how to improve my code is appreciated.
var message ='';
var student;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = "";
outputDiv.innerHTML = message;
}
function getIndexValue(search){
var studentName;
for (var x=0; x < students.length; x+=1){
studentName= students[x].name.toLowerCase();
if( studentName === search) {
return x;
}
}
return -1;
}
function Itemfound(index) {
if (index > -1) {
student = students[index];
message = '<h2>Student Name : ' + student.name + '</h2>';
message+= '<p>Track : ' + student.track + '</p>';
message+= '<p>Achievements : ' + student.achievements + '</p>';
message+= '<p>Points : ' + student.points + '</p><br>';
} else {
message = 'Search not found in the student list';
}
print(message);
}
while(true){
search = prompt("Search for a student. Type 'quit' to exit");
if (search === 'quit') {
break;
} else {
var searchlowercase = search.toLowerCase();
var IndexValue = getIndexValue(searchlowercase);
Itemfound(IndexValue);
}
}
1 Answer
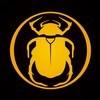
rydavim
18,814 PointsI think your code is fine as it is, and certainly easier to read than using indexOf. However, if you wanted to use indexOf in order to eliminate the getIndexValue function, you could do so using something like the code below.
while(true){
search = prompt("Search for a student. Type 'quit' to exit");
if (search === 'quit') {
break;
} else {
// The students.map function returns an array of the student names.
// Then you can use indexOf with some string manipulation in order to search those names and get an index for the object.
var indexValue = students.map(function(e) { return e.name; }).indexOf(search.charAt(0).toUpperCase() + search.toLowerCase().slice(1));
itemFound(indexValue);
}
}
I think in terms of efficiency though, this is probably a worse solution. To use indexOf as above, you have to iterate over the entire array. In your solution, you may find the index you're looking for before iterating over the whole thing.
My only suggestion going forward would be to make sure you're being consistent in your naming conventions.
// Your variables and function names use several different naming patterns.
var searchlowercase; // all lowercase
var IndexValue; // camelcase with capital letter start
function getIndexValue(search) { }; // camelcase with lowercase letter start
There are no hard and fast rules about how you should name things, but it is best practice to pick a convention and stick to it. I'm partial to camelcase with a lowercase start.
You're doing great, keep on coding! :)
vanessa
3,455 Pointsvanessa
3,455 PointsThanks Rydavim! will try your code & follow your advise. Didn't notice my naming patterns aren't the same. Thanks for pointing out.