Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial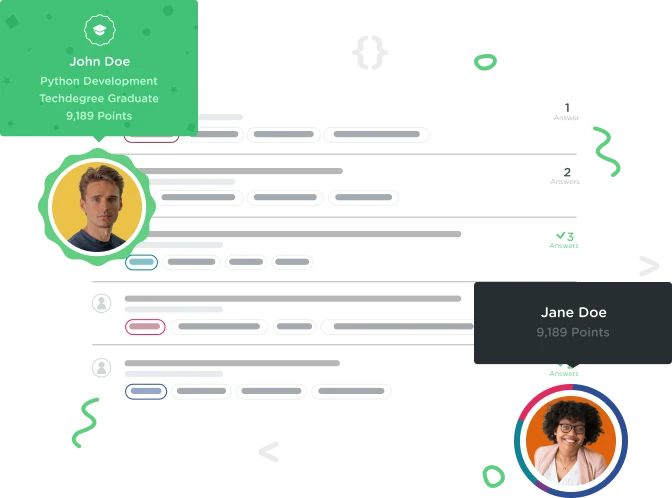

Ryan Holdeman
8,067 PointsWhat am I doing wrong?
I'm trying to change my "while" loop to a "do while" loop and I'm not understanding how I'm getting it wrong. I'm replacing the while loop at the beginning with a "do" and putting that while loop at the end. Thank you
var secret = prompt("What is the secret password?");
do {
secret = prompt("What is the secret password?");
} while ( secret !== "sesame" )
document.write("You know the secret password. Welcome.");
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers
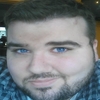
Marcus Parsons
15,719 PointsHey Ryan,
You are so very close. The "problem" here is redundancy. It is a good idea to set the "secret" variable equal to a prompt when you are doing a while loop because the condition is checked before the code is executed and if a user guesses the secret right off the bat, the while loop has no need to execute and the document.write() will fire off.
With a do while loop, however, the code block is always executed before the condition is checked (opposite of while loop). So, in the case of the do while, all that is necessary is to just create the "secret" variable but not set it to a prompt immediately. Because if you set the variable "secret" to a prompt outside of the loop when you create it, and then set it again to a prompt inside the loop, you'll see a prompt asking you for the secret, and then another one right after that, because it is executing the creation of the variable with a prompt, and then immediately going to the do while and executing another prompt. Then it checks the condition to see if "secret" equals "sesame", but it only checks the 2nd value you put in because the first one is overwritten by the 2nd prompt.
Copy and paste your code into your console and you'll see what I mean about the double prompt.
Here is all you need to pass the challenge (just do away with the first prompt):
var secret;
do {
secret = prompt("What is the secret password?");
} while ( secret !== "sesame" )
document.write("You know the secret password. Welcome.");

Niclas Valentiner
8,947 PointsMarcus seems to have forgotten to mention that what you're checking is case sensitive. If a user would give the input of "Sesame" it would be incorrect, as would "SESAME" or any other spelling than all lower case.
//This fixes your issue because it converts the value in secret
// to all lower case characters then compares with all lower case characters
while ( secret.toLowerCase() !== "sesame" )
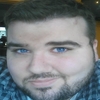
Marcus Parsons
15,719 PointsYou would want your secret to be case sensitive so that it is harder to guess, so I would advise against transferring the capitalization to lower or upper case. That's why I did not include it. Why make the secret easier to guess? LOL

Niclas Valentiner
8,947 PointsNot disagreeing but because it seemed to me like he has/had a problem with the loop, and a part of that is breaking out of the loop. Just wanted to clarify how he's supposed to do that (either being case sensitive in his input or making the check case insensitive).
As for passwords and actual secrets, I agree that there's no point to going case insensitive.
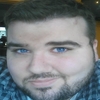
Marcus Parsons
15,719 PointsThe problem wasn't breaking out of the loop, Niclas. This is for a challenge and the challenge did not want you to put a prompt in the creation of the var "secret", because it results in a double prompt displaying. It doesn't matter what you put in the first prompt because it is overwritten by the 2nd prompt that exists in the do while code block.
And in a normal environment, I always do lower case or upper case conversions. But, as I said, this is for a secret password and therefore should not be used with a capitalization conversion method (although you can still pass the challenge with it).