Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial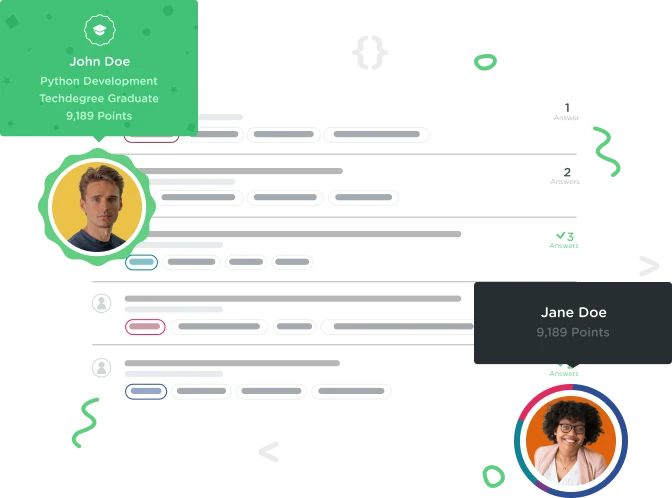
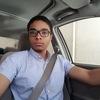
Luqman Shah
3,016 PointsWhat am I doing wrong?
On the js console it says SASUKE is undefined on line 5, and when I set the answers as string values instead like "Sasuke," and tested out the code in the browser, only the else program would run, even when I put the correct answer. Also, the document.write message at the end won't print to the webpage, why?
var questions = 5;
var correctAns = 0;
var questionOne = prompt("Who is Naruto's rival?");
if ( parseInt(questionOne) === SASUKE ) {
alert('You got it!');
correctAns +=1;
} else {
alert('Oops, wrong answer');
correctAns -=1;
}
var questionTwo = prompt("Who is Sasuke's rival?");
if ( parseInt(questionTwo) === NARUTO ) {
alert('You got it!');
correctAns +=1;
} else {
alert('Oops, wrong answer');
correctAns -=1;
}
var questionThree = prompt("Who is Sakura's crush?");
if ( parseInt(questionThree) === SASUKE ) {
alert('You got it!');
correctAns +=1;
} else {
alert('Oops, wrong answer');
correctAns -=1;
}
var questionFour = prompt("Who is Hinata's crush?");
if ( parseInt(questionOne) === NARUTO ) {
alert('You got it!');
correctAns +=1;
} else {
alert('Oops, wrong answer');
correctAns -=1;
}
var questionFive = prompt('What show are we talking about?');
if ( parseInt(questionFive) === NARUTO ) {
alert('You got it!');
correctAns +=1;
} else {
alert('Oops, wrong answer');
correctAns -=1;
}
if ( correctAns === 5 ) {
document.write("Congrats, you've earned the gold crown");
}
if ( correctAns === 4 ) {
document.write("Congrats, you've earned a silver crown");
}
if ( correctAns === 3 ) {
document.write("Congrats, you've earned a silver crown");
}
if ( correctAns === 2 ) {
document.write("Congrats, you've earned a bronze crown");
}
if ( correctAns === 1 ) {
document.write("Congrats, you've earned a bronze crown");
}
if ( correctAns === 0 ) {
document.write("Sorry, you lose..");
}
1 Answer
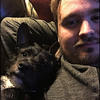
Matthew Long
28,407 PointsYou're correct that you need to compare the values as strings. This means you don't need to convert them to an integer like you're doing. And you need to put the answers between quotes like "SASUKE"
. It might also be a good idea to use .toLowerCase()
on the prompt and then make the answers "sasuke"
. This way "SASUKE"
, and "SaSukE"
, etc are correct.
Luqman Shah
3,016 PointsLuqman Shah
3,016 PointsThanks! so I gave the whole program another go with different questions, and I tried your suggestion:
But when I type in Trump, it runs the else program
Luqman Shah
3,016 PointsLuqman Shah
3,016 PointsOhhh nvm on the prompt itself, gotcha lol sorry
Matthew Long
28,407 PointsMatthew Long
28,407 PointsTry this:
Luqman Shah
3,016 PointsLuqman Shah
3,016 PointsI actually tried this: and it worked :)
Thank you though, this was a real help! Is this also sufficient?