Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial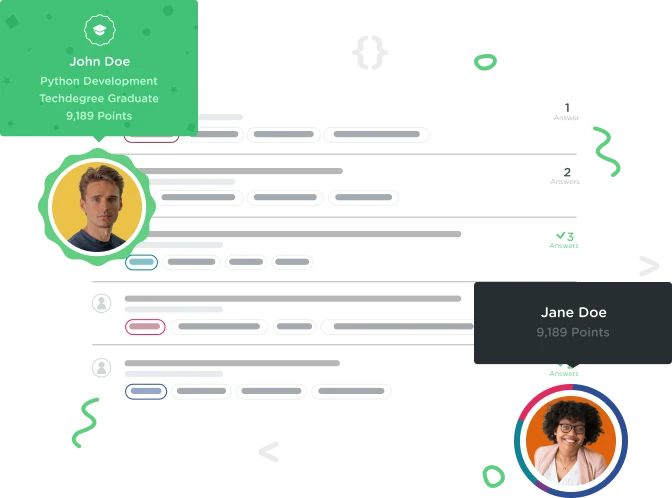

Chetan Jaisinghani
2,896 PointsWhat am I doing wrong here?
In this video, Dave created a function and the for loop was then modified so that values for red, blue and green each come from calling the function. Instead of:
red = randomRgb ();
I want to change it in a way that I can pass arguments to parameters in the function like this:
randomRgb(red);
What am I doing wrong here?
var html = '';
var red;
var green;
var blue;
var rgbColor;
var color;
function colorBlocks(color){
color = Math.floor(Math.random() * 256 );
return color;
}
for (var i = 0; i < 10; i += 1) {
colorBlocks(red);
colorBlocks(green);
colorBlocks(blue);
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
html += '<div style="background-color:' + rgbColor + '"></div>';
}
document.write(html);
2 Answers
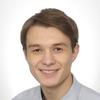
Sergey Podgornyy
20,660 PointsYou don't need to send any parameters to function. If you want to generate color, just create variables, like in code below
var html = '', red, green, blue, rgbColor;
function colorBlocks(){ return Math.floor(Math.random() * 256 ); }
for (var i = 0; i < 10; i ++) {
red = colorBlocks();
green = colorBlocks();
blue = colorBlocks();
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
html += '<div style="background-color:' + rgbColor + '; height:50px; width:100%"></div>';
}
document.write(html);
May the Force be with you, jung Padawan ;)
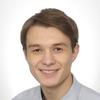
Sergey Podgornyy
20,660 PointsOr you can do it without creating variables:
var html = '';
function colorBlocks(){ return Math.floor(Math.random() * 256 ); }
for (var i = 0; i < 10; i ++) {
html += '<div style="background-color: rgb(' + colorBlocks() + ',' + colorBlocks() + ',' + colorBlocks() + '); height:75px; width:100%"></div>';
}
document.write(html);
Chetan Jaisinghani
2,896 PointsChetan Jaisinghani
2,896 PointsThis is what was shown in the video. However, my question was, how can I 'change' it in order for it to work the way I want it to (i.e. by sending arguments to parameters)?
Sergey Podgornyy
20,660 PointsSergey Podgornyy
20,660 PointsYou don't need to send arguments, this function return value. What do you mean change it in order? RGB model always take Red first, Green second and Blue third, that why it calls RGB model