Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial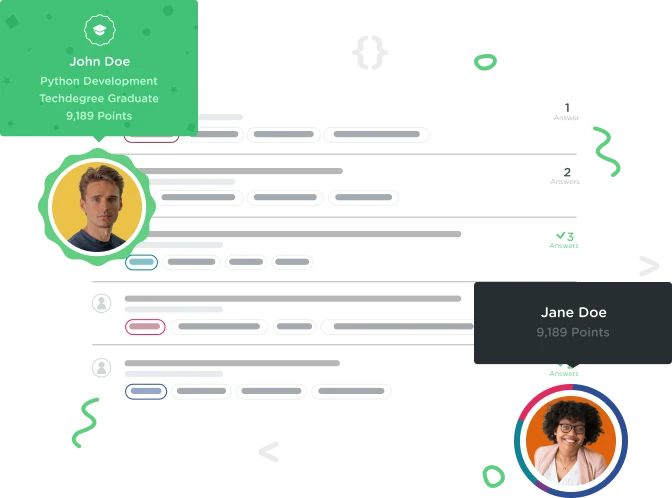
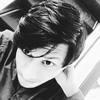
Mit Sengupta
13,823 PointsWhat am I doing wrong here? Do I need to call the even_odd function in the while loop?
Here is my code :
import random
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
start = 5
while True:
start = random.randint(1, 99)
for num in start:
if num == None:
break
elif num % 2 == 0:
print("{} is even".format(num))
elif num not % 2 == 0:
print("{} is odd".format(num))
start -= 1
3 Answers
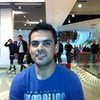
Andreas cormack
Python Web Development Techdegree Graduate 33,011 PointsHi Mit
Yes you need to call the even_odd function, you also dont need a for loop. Break the while loop when start becomes 0 or falsey.
import random
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
start = 5
while True:
rand_number = random.randint(1,99)
if start == 0:
break;
elif even_odd(rand_number):
print("{} is even".format(rand_number))
else:
print("{} is odd".format(rand_number))
start-=1
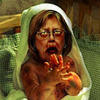
Johnathan Young
Courses Plus Student 1,573 Pointshey Justo. I was having the same issue. Turns out its the periods we have at the end of our "is even." and "is odd." statements. If you remove those ("is even" and "is odd") it will work perfectly.

Sharla Kew
15,356 PointsSweet lord, I thought I was losing my mind. Thank you.
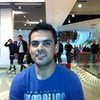
Andreas cormack
Python Web Development Techdegree Graduate 33,011 PointsHi Justo
I ran your code in workspaces and it runs perfectly .
98 is even.
16 is even.
51 is odd.
2 is even.
59 is odd.
Your understanding is correct, There might be a bug in the challenge, may be Kenneth Love can help here.
Justo Montoya
3,799 PointsJusto Montoya
3,799 Pointshey i'm having a bit of trouble with this too. my code is :