Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial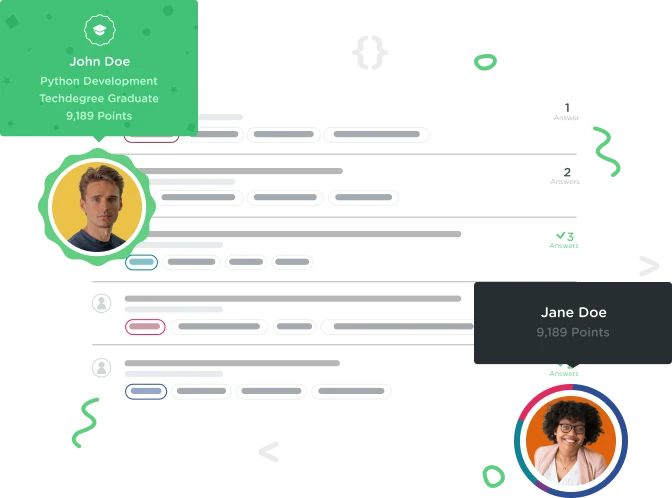
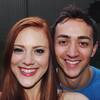
Tyler Herbst
11,763 PointsWhat am I missing here?
Everything seems to work fine except that it's printing the "Sorry, there is no student..." message for every search.
For example, if I have two "Jodys," it will print all of the information for both Jodys, but it will also say "Sorry..."
Thank you!
var message = '';
var student;
var search;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport( student ) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
search = prompt('Search student records: type a name [Jody] (or type "quit" to end)');
if (search === null || search.toLowerCase() === 'quit') {
break;
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (student.name.toLowerCase() === search.toLowerCase() ) {
message += getStudentReport( student );
} else if (i === students.length - 1){
message += '<p>Sorry, there is no student registered by the name of ' + search + '</p>';
}
}
print(message);
}
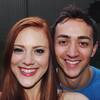
Tyler Herbst
11,763 PointsHi Ira,
Thanks for the reply! I should have noted that there is another js file where the "students" array is defined. You have a good eye!
Thanks, Tyler
3 Answers
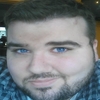
Marcus Parsons
15,719 PointsHey Tyler,
Just to let you know, the problem does not lay within any kind of undefined array as that is defined in a separate js file. The reason why it is printing out the "Sorry..." statement along with the student information is that i
will become equal to students.length - 1
by the end of the iteration of the loop always. This means that every time you execute your loop, that else if
statement executes.
So, instead of using that else if
there, I actually turned it into an if-statement inside the for
loop to check to see if we are at the end of the loop and a student has not been found. If a student wasn't found in the array, it will return the "Sorry..." message like so:
while (true) {
search = prompt('Search student records: type a name [Jody] (or type "quit" to end)');
//Added studentFound variable
var studentFound = false;
if (search === null || search.toLowerCase() === 'quit') {
break;
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (student.name.toLowerCase() === search.toLowerCase() ) {
message += getStudentReport( student );
//If a student is found, mark this variable as true
studentFound = true;
}
//Check to see if we are at the end of the loop and a student has not been found
if (i === students.length - 1 && studentFound !== true) {
message += '<p>Sorry, there is no student registered by the name of ' + search + '</p>';
}
}
print(message);
}
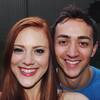
Tyler Herbst
11,763 PointsThank you Marcus!
That makes perfect sense. I'm sure I'll be asking you for more help in the future.
Thanks, Tyler
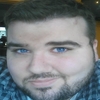
Marcus Parsons
15,719 PointsAnytime, Tyler! You can shoot me an email at marcus.parsons@gmail.com or just tag me on here.
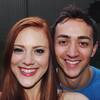
Tyler Herbst
11,763 PointsSounds good! Thanks again!
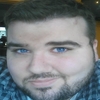
Marcus Parsons
15,719 PointsNo problem, man haha
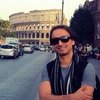
Henrique Machuca
5,611 PointsI found that in your code when you search for a name that has duplicates it shows up both, but when you search again, the results will add up to the previous. They will never reset.
Did you notice that?
Just add the sentence below at the end of the code, below print(message);
message = ' ';
This way this variable will reset, and when you search again and a match is stored in it, it wonΒ΄t add up with previous results.
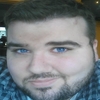
Marcus Parsons
15,719 PointsHey Henrique,
The problem with that is that if you clear the message
variable each time, you will erase the previously looked up records, which is not ideal. There are no duplicates in the students array unless you add them, as well.
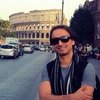
Henrique Machuca
5,611 PointsItΒ΄s true.
But for the sake of an example, lets say I add a duplicate name.
With the original code, it works cause it lists me both names and information. The problem is that when I search again for another student name, the information will stack up with the previous one and will keep showing me my 2 previous matching results plus my lasted search, and so on.
How can I fix this in a better way?
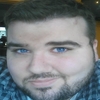
Marcus Parsons
15,719 PointsThat's the point of the program. Every time you search for a student, it adds that student's information to the page. But to eliminate showing duplicates, you can just add an and (&&) statement to the first if-statement that checks to see if studentFound is not true, and if it is not and the search matches, then it will get the report for the student searched:
if (student.name.toLowerCase() === search.toLowerCase() && studentFound !== true) {
message += getStudentReport( student );
//If a student is found, mark this variable as true
studentFound = true;
}
Ira Bradley
12,976 PointsIra Bradley
12,976 PointsI believe the problem is that you never defined the array "students". You for loop is attempting to iterate over an array that doesn't exist.