Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial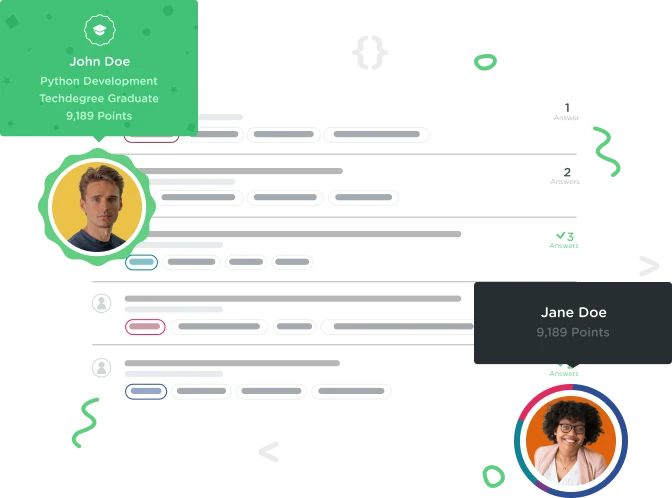

Kevin Caruso
3,930 PointsWhat am i missing on this challenge?
I thought i could change it so the values in the dictionary were the len(value) so the dictory would read { teacher: int} then i broke the dictionary into tuples so id have ordered lsit and then append them into my empty list.
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(dict):
teacher_count = 0
for teacher in dict:
teacher_count +=1
return teacher_count
def num_courses(dict):
courses = 0
for value in dict.values():
courses += len(value)
return courses
def courses(dict):
new_list = []
for value in dict.values():
new_list.extend(value)
return new_list
def most_courses(dict):
winning_teacher = ''
max_number = 0
for key, value in dict.items():
if len(value) > max_number:
max_number = len(value)
winning_teacher = key
return winning_teacher
def stats(dict):
tuple_list = []
for value in dict.values():
value = len(value)
for item in dict.items():
tuple_list.append(item)
return tuple_list
1 Answer
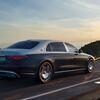
Balazs Peak
46,160 PointsYou have mixed things up a little bit. I wouldn't bother commenting on it, it seems like you've just got tired and mixed stuff up.
As I demonstrate here, you need to create a list of lists. This list consists of 2 item lists, the first is the name, the second is the number of courses. In the dictinionary, the key is responsible for the name, the courses' list can give you the number of courses, if you use the "len()" function.
Keep coding!
def stats(dict):
tuple_list = []
for key, value in dict.items():
tuple_list.append([key, len(value)])
return tuple_list