Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial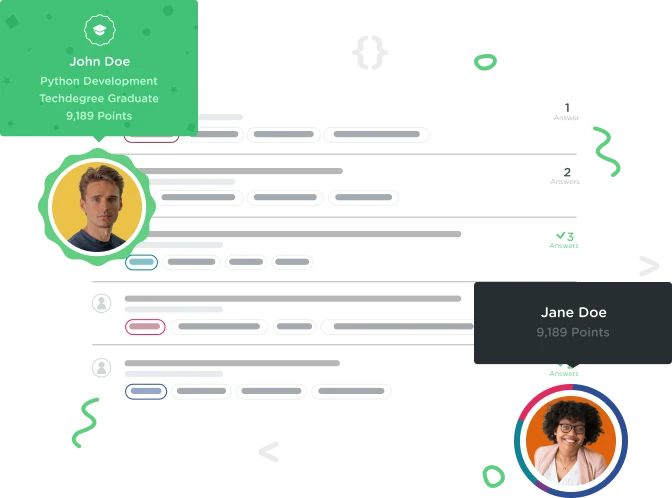

MUZ140884 Maxwell Makumucha
6,291 PointsWhat code must I write so that the alert message "Its Friday,but i don't have enough money to go out"
var money = 9; var today = 'Friday'
if ( money >= 100 || today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 || today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 || today === 'Friday' ) {
alert("Time for a movie");
} else if ( today !== 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
var money = 9;
var today = 'Friday'
if ( money >= 100 || today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 || today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 || today === 'Friday' ) {
alert("Time for a movie");
} else if ( today !== 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers
John Steer-Fowler
Courses Plus Student 11,734 PointsHi,
You need to adjust multiple statements in this challenge.
Your code should look something like:
var money = 9;
var today = 'Friday'
if ( money >= 100 && today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 && today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 && today === 'Friday' ) {
alert("Time for a movie");
} else if ( money < 10 && today === 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
Read through this code line by line and see why this makes sense. We have a unit of 9 money and today is Friday.
The code is now saying:
- If we have 100 or more money AND today is Friday then lets go to the theater.
- If we have 50 or more money AND it's Friday lets go for a movie and dinner
- If we have more than 10 money AND it's Friday, lets go for a movie
- If we have less than 10 money AND it's Friday, then we don't have enough money to go out
- Otherwise, it's not Friday and we stay home.
I hope this helps
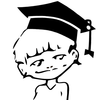
simhub
26,544 Pointshi Maxwell, it is like John said. with this in mind, you could do something like this:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Money</title>
</head>
<body>
<label for="m">money</label>
<input type="text" id="m" value="9"/>
<label for="today">day</label>
<input type="text" id="today">
<button id="money">answer</button>
<script>
var answer = {
day: "friday",
one: "Time to go to the theater",
two: "Time for a movie and dinner",
three: "Time for a movie",
four: "This isn't Friday. I need to stay home.",
five: "It's Friday, but I don't have enough money to go out",
whatDay: function (d) {var day = d.toLowerCase();return day === this.day;}};
var m = document.getElementById("money");
m.addEventListener("click", function () {
var money = document.getElementById("m").value;
var today = document.getElementById("today").value;
today = today.toLowerCase();
if (money >= 100 && answer.whatDay(today)) {
alert(answer.one);
} else if (money >= 50 && answer.whatDay(today)) {
alert(answer.two);
} else if (money >= 10 && answer.whatDay(today)) {
alert(answer.three);
} else if (!answer.whatDay(today)) {
alert(answer.four);
} else {
alert(answer.five);
}
});
</script>
</body>
</html>