Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial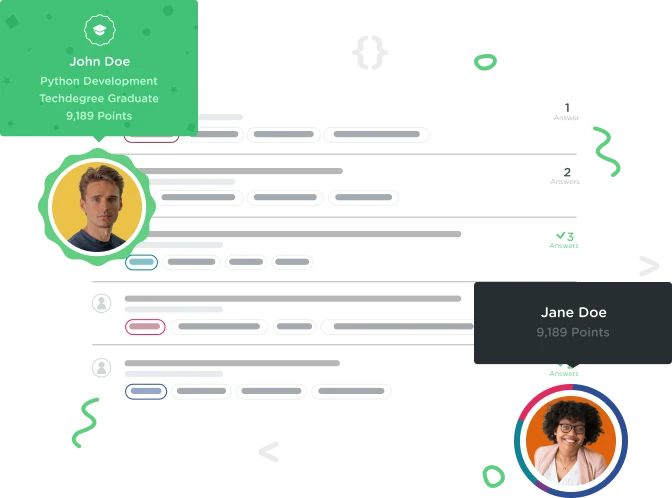

Musah ali
528 Pointswhat do i do here?
help pls
available = "banana split;hot fudge;cherry;malted;black and white"
1 Answer
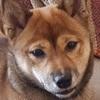
Katie Wood
19,141 PointsHi there,
Let's go through it step by step. First, it wants you to split that long string into individual flavors, in a variable called sundaes. This is where our .split() comes in. We can see that the flavors are separated by semicolons, so that is what we pass into the split method:
available = "banana split;hot fudge;cherry;malted;black and white"
sundaes = available.split(';')
That's Step 1 done. So, sundaes now contains something that looks like ['banana split', 'hot fudge', 'cherry', 'malted', 'black and white'].
The next step wants us to write a new string. This one is pretty straightforward - just make the new variable and set it to what the challenge says:
available = "banana split;hot fudge;cherry;malted;black and white"
sundaes = available.split(';')
menu = "Our available flavors are: {}."
That's Step 2 done.
In Step 3, it gives you two options to solve it. You can either combine sundaes into a new variable and use that to format the menu string, or you can do it all in one line without the new variable. The new variable is to be called display_menu, so let's look at what both of those should look like.
With the variable:
Since we're going to use .format() on the menu variable with this new string, we need the string to be declared before menu. We need to join the sundaes variable into one string, separated by commas. Here's how we do that:
available = "banana split;hot fudge;cherry;malted;black and white"
sundaes = available.split(';')
display_menu = ', '.join(sundaes)
menu = "Our available flavors are: {}." #don't copy-paste this one - we're not done yet!
The display_menu variable now contains a string that looks like "banana split, hot fudge, cherry, malted, black and white". This is what we want in our menu variable, so let's make that appear where the {} are using .format:
available = "banana split;hot fudge;cherry;malted;black and white"
sundaes = available.split(';')
display_menu = ', '.join(sundaes)
menu = "Our available flavors are: {}.".format(display_menu)
That's one way to do it, and if you want, you're done here.
The "bonus objective" to do it in one line would be very similar to this, though - you just bypass using display_menu at all and just pass the join() into the .format(), like this:
available = "banana split;hot fudge;cherry;malted;black and white"
sundaes = available.split(';')
menu = "Our available flavors are: {}.".format(', '.join(sundaes))
And there we go - hope this helps!