Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial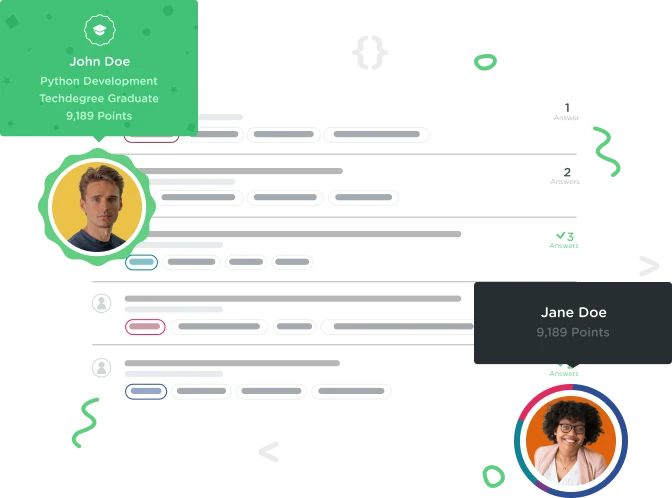

ziv brodie
1,348 PointsWhat do you mean when you say "Interfaces"?
When you keep on mentioning Interfaces, are you referring to the type of class that must be implemented by a different class along with its abstract methods, or is it something entirely different?
1 Answer

Jon Baum
13,863 PointsInterfaces and abstract classes have a lot in common. The key differences are that:
Abstract classes are more expensive (more cpu time, more memory) than interfaces in most object oriented languages (like Java).
Abstract classes can implement functionality (methods and fields) in itself. Interfaces are blueprints only and can include no functionality, only method signatures.
You can implement multiple interfaces in a single class, but can only extend one abstract class.
The way I view the difference between them is to imagine an abstract class is a more general classification of some classes I designing. That is if I'm building three classes Dog, Cat and Goldfish I might abstract some common functionality like a getName, getAge or getWeight method into an abstract class Pet and include the code for those methods in Pet so I don't have to write it in each of the three child classes. So imagine the following example:
abstract class Pet {
private String name;
private int age;
private int weight;
public String getName() {return name;}
public int getAge() {return age;}
public int getWeight() {return age;}
}
public class Dog extends Pet {
}
public class Cat extends Pet {
}
public class Goldfish extends Pet {
}
Now the benefit of abstract classes over a simple parent class is those abstract methods we can specify for implementation in the child classes:
abstract class Pet {
private String name;
private int age;
private int weight;
public String getName() {return name;}
public int getAge() {return age;}
public int getWeight() {return age;}
public abstract String getSaying();
}
public class Dog extends Pet {
public String getSaying(){return "Woof";}
}
public class Cat extends Pet {
public String getSaying(){return "Meow";}
}
public class Goldfish extends Pet {
public String getSaying() {return "Bubble noises";}
}
Interfaces on the other hand can provide NO implementation details. So using the above example with a Interface we get the following instead:
public interface Pet {
public String getName();
public int getAge();
public int getWeight();
public abstract String getSaying();
}
public class Dog implements Pet {
private String name;
private int age;
private int weight;
public String getName() {return name;}
public int getAge() {return age;}
public int getWeight() {return age;}
public abstract String getSaying() {return "Woof";}
}
public class Cat implements Pet {
private String name;
private int age;
private int weight;
public String getName() {return name;}
public int getAge() {return age;}
public int getWeight() {return age;}
public String getSaying(){return "Meow";}
}
public class Goldfish implements Pet {
private String name;
private int age;
private int weight;
public String getName() {return name;}
public int getAge() {return age;}
public int getWeight() {return age;}
public String getSaying() {return "Bubble noises";}
}
As you can see we get three times the code bloat and have to change things multiple times. This hopefully shows that abstract classes should be used when you know you have functionality in common.
Interfaces are extremely useful for cases where we want to use a class in the same way but don't want to have to worry about how its implemented. This applies to cases like testing. Imagine we are trying to test GPS functionality in an app that also stores that GPS data in a database for later. We shouldn't have to actually store the data in the database in order to test the fact that the GPS components of the app are working. We should be able to pretend to store the data without actually wasting all the time of creating a new database, storing the data, validating its storage, destroying the data and the database etc all to just test "Do I setup to get a GPS signal properly?". We can mock up the database in this context using interfaces Database with implemeting classes RealDatabase and FakeDatabaseForTesting without having to have ANY functionality with the actual database in common. This is a common use of dependency injection.
Now that being said. There is an example of a PURE abstract class: (no fields, no actual methods, just abstract methods).
public abstract Pet {
public abstract String getName();
public abstract int getAge();
public abstract int geWeight();
public abstract String getSaying();
}
These are very similar to interfaces except for #1,3 mentioned above. Firstly, while most premature optimization should be avoided, the use of a pure abstract class in the place of an interface is just a poor choice and a sign of code smell. Now there may be some weird corner cases, but in most cases you should use an interface instead of a pure abstract class. Secondly, interfaces allow you do to use multiple interfaces on a single class:
public interface Serializable {
public String getString();
}
public interface ConsoleOutput {
public void outputToConsole();
}
public interface Pet {
public String getName();
public int getAge();
public int getWeight();
public abstract String getSaying();
}
public class Dog implements Serializable, Pet {
private String name;
private int age;
private int weight;
public String getName() {return name;}
public int getAge() {return age;}
public int getWeight() {return age;}
public abstract String getSaying() {return "Woof";}
public String getString(){name + ": " + age + ", " + weight};
}
public class OutputCounter implements Serializable, ConsoleOutput {
private int counter;
public String getString{) {
counter += 1;
return "The count: " + counter;
}
public void outputToConsole() {
counter = counter + 1;
System.out.prinln("" + counter);
}
}
As you can see we can mix and match interfaces to the needs of our classes, picking up certain functionality when we need to and ignoring it when we don't. The major disadvantage of pure abstract classes is that we can only extend ONE abstract class. We can implement a stupid amount of interfaces. For more reading on why you can extend multiple interfaces, the main cause is the diamond problem.
Hopefully this clears up when to use abstract classes and when to use interfaces. As a TL:DR Use abstract classes when you have methods that are going to do the exact same thing in the exact same way in different classes and use interfaces when you are going to do similar things but in possibly different ways.
I wrote this in a hurry so let me know if any of the code/spelling is wrong. Thanks!