Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial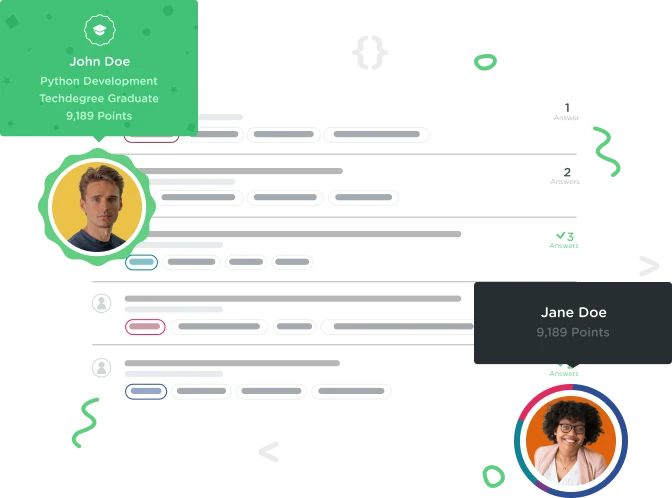

boo park
1,169 PointsWhat does it mean when I get the message "too many values to unpack (expected 2)"
I'm on the last code challenge of the Dictionary for python. I finished the challenge and passes it, but just in case, I tried it again. This time my code didn't go through so smoothly and I get the following message:
"too many values to unpack (expected 2)"
What does it mean exactly? I can't be too far off from the correct answer since I already passed this challenge with the similar code.
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
def most_classes(teacher):
max_count = 0
busy_teacher = ' '
for k, v in teacher.items():
if len(v) > max_count:
max_count = len(v)
busy_teacher = k
return busy_teacher
def num_teachers(teachers):
return len(teachers)
def stats(teachers):
teacher_name = ' '
num_classes = 0
teacher_class_num = []
for teacher, classes in teachers.items():
teacher_name = teacher
num_classes = len(classes)
teacher_class_num.append([teacher_name, num_classes])
return teacher_class_num
def courses(teachers):
courses = []
teacher_classes = []
for teacher, classes in teachers:
for each_class in classes:
teacher_classes.append(each_class)
for one_class in teacher_classes:
if one_class in courses:
continue
else:
courses.append(one_class)
return courses
2 Answers
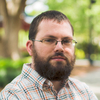
Kenneth Love
Treehouse Guest TeacherIt's this line: for teacher, classes in teachers:
that's causing it. teachers
is a dict and can't be unpacked into two variables like you've tried to do.
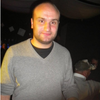
Vittorio Somaschini
33,371 PointsHello boo park,
I think this is way too much code for this challenge, and honestly I can't explain that error, but there is a handy method in python that you can use here, "values()", so:
def courses(teachers):
courses_list = []
for course in teachers.values():
courses_list.extend(course)
return courses_list
values() works like items() that you have used before but returns the values this time, a bit more info here http://www.tutorialspoint.com/python/dictionary_values.htm
At the end we use extend and not append, please have a quick look here (it explains why): http://stackoverflow.com/questions/252703/python-append-vs-extend
;)