Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial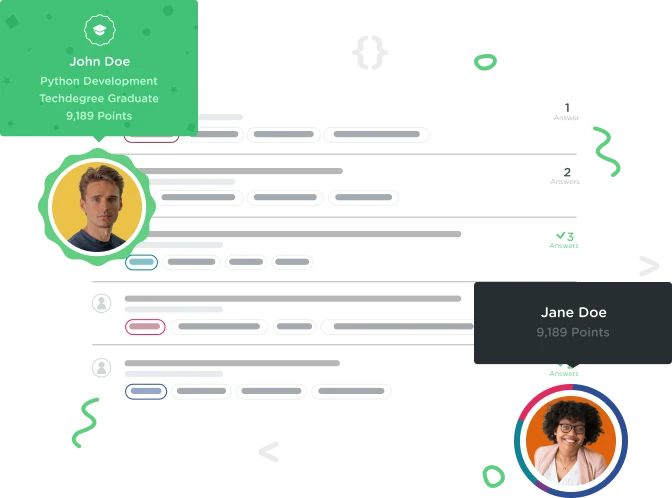

SEUNG IL YANG
1,035 PointsWhat does the output look like?
"""Wow, I just can't stump you! OK, two more to go. I think this one's my favorite, though. Create a function named most_courses that take our good ol' teacher dictionary. most_courses should return the name of the teacher with the most courses. You might need to hold onto some sort of max count variable."""
I have run into the question that I can not understand how to start off.
I would like to know what it does mean by "The most courses" in the context above?
Could anyone help out the poor Korean man unable to digest what this question want from me?
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(args):
return len(dict(args).keys())
def num_courses(b):
return sum(len(v) for v in b.values())
def courses(args):
Lis = []
for v in args.keys():
for x in args[v]:
Lis.append(x)
return Lis
8 Answers

Parsenz Dev
13,198 PointsIt means that you need to return the teacher from the dict who has the longest list of courses
def most_courses(teacher_dict):
# Initialize a counter to track the teacher with the highest number of courses
highest_tally = 0
rockstar = ""
# For each teacher and their list of courses, compare to the current longest length of courses
for teacher, course_list in teacher_dict.iteritems():
if len(course_list) > highest_tally:
# If this person teaches more than our current rockstar, then record
# this teacher as the rockstar and set the highest tally to the length of their course list
highest_tally = len(course_list)
rockstar = teacher
return rockstar

Allison Schaaf
33,322 PointsMine is basically the same as Parsenz Dev's code but change .iteritems() to .items()
def most_courses(teacher_dict):
max_count = 0
rockstar = ""
for teacher, course_list in teacher_dict.items():
if len(course_list) > max_count:
max_count = len(course_list)
rockstar = teacher
return rockstar
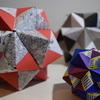
Hope Watson
5,496 PointsI like this one more since it is sticking to what we have learned over the courses instead of adding in code that hasn't been taught yet. Thank you.

Abubakar Gataev
2,226 PointsI don't really get this one, how do you know that 'teacher' has the most courses?

Parsenz Dev
13,198 Points@youssef moustahib
A somewhat simplified explanation; assume you have a dict of teachers and their subjects like the following:
In [1]: teachers = {'Mark': ['Music'], 'Bob': ['Math', 'Science', 'Gym']}
the items method will give you a copy of the dictionary's list of tuples of keys to values as shown below:
In [2]: teachers.items()
Out[2]: [('Bob', ['Math', 'Science', 'Gym']), ('Mark', ['Music'])]
iterating over the return of the iteritems method however will give you the same result, except internally it's optimized for performance by returning an iterator (not a copy) over the dictionary's list of key value tuples as demonstrated below:
In [4]: teachers.iteritems()
Out[4]: <dictionary-itemiterator at 0x7f0ce10c69f0>
In [5]: for teacher, subjects in teachers.iteritems():
print(teacher, subjects)
Out[5]: ('Bob', ['Math', 'Science', 'Gym'])
('Mark', ['Music'])
Hope that helps.
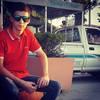
Robert Stepanyan
2,297 Pointsdef most_courses(dictionary):
new_list = {}
max = 0;
for key, value in sorted(dictionary.items()):
new_list[key] = len([item for item in value if item])
current_number = new_list[key]
if current_number > max:
max = current_number
max_key = key

Abubakar Gataev
2,226 PointsCan't you solve this one with .keys() just wanna know that because i tried it with .keys() and it didn't work? please answer A.S.A.P.

James Montgomery
5,182 PointsNot sure why this is returning "Bummer, try again".
def most_courses(items):
max_course = 0
teacher = ""
for k, v in items.items():
if len(v) > max_course:
max_course = len(v)
teacher = k
return teacher

Vlad Bitca
Courses Plus Student 2,702 PointsMy short version
def most_courses(dict):
return(max(dict.keys(), key=lambda x: len(dict[x])))

David Toops
19,151 PointsThis is long but it captures all teachers that have the same max number of courses
def most_courses(teach_dict):
teacher_list = []
max_cnt = 0
cur_cnt = 0
for key in teach_dict.keys():
cur_cnt = len(teach_dict[key])
if cur_cnt > max_cnt:
teacher_list = [key]
max_cnt = cur_cnt
elif cur_cnt == max_cnt:
teacher_list.append(key)
if len(teacher_list) == 1:
return teacher_list[0]
else:
return teacher_list
SEUNG IL YANG
1,035 PointsSEUNG IL YANG
1,035 PointsThanks for your comment. :>
Shadow Skillz
3,020 PointsShadow Skillz
3,020 PointsHey parsenz just was curious what the method iteritems does exactly. I'm also not understating how 2 for loop variables can differ from each other. I understand what you wrote, but i still need more clarity in that area so I can begin to implement that technique myself.
Ashish Shah
1,468 PointsAshish Shah
1,468 PointsThis does not work
youssef moustahib
2,303 Pointsyoussef moustahib
2,303 PointsHi Parsenz,
What does this line mean, I don't think i've come across it so far in my python journey --->
for teacher, course_list in teacher_dict.iteritems():
I haven't seen that way of writing a for loops before..
Terry Felder
1,738 PointsTerry Felder
1,738 Pointsi tired your code and went to pass it and it failed....i dont understand why! the code makes sense to me..i keep having this problem. is treehouse looking for a specific answer or what??
im to the point where i cant wait to move on from dictionaries..my codes run perfectly fine but treehouse makes me look up other peoples codes and use them as answers..
Eric Harris
2,391 PointsEric Harris
2,391 PointsIn Parsenz Dev example the code below loops over the keys in the dictionary. Had to look this up. Stack overflow link below:
for teacher, course_list in teacher_dict.iteritems():
https://stackoverflow.com/questions/3294889/iterating-over-dictionaries-using-for-loops