Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial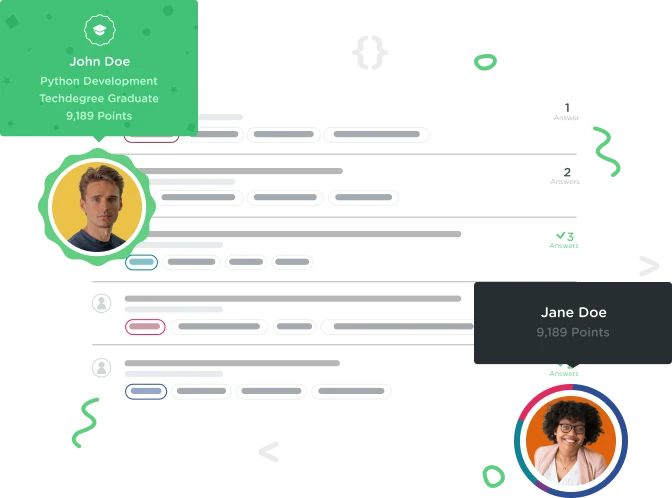

Alex Avila
8,570 PointsWhat is a simpler way to do the teacher stats code challenge?
I resisted the urge to google the answers and this is what I came up with. However, I feel like there's a much simpler way to do these functions. Especially the most_courses function.
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(d):
return len(d.keys())
def num_courses(d):
total_courses = []
for value in d.values():
for val in value:
total_courses.append(val)
return len(total_courses)
def courses(d):
total_courses = []
for value in d.values():
for val in value:
total_courses.append(val)
return total_courses
def most_courses(d):
courses_num_list = []
teachers_list = []
indexes = []
all_indexes = []
most_teachers = []
for courses in d.values():
courses_num = len(courses)
courses_num_list.append(courses_num)
for teachers in d.keys():
teachers_list.append(teachers)
count = 0
for num in courses_num_list:
if count == 0:
high_val = num
count += 1
else:
if num > high_val:
high_val = num
else:
pass
indexes = [index for index, value in enumerate(courses_num_list) if value == high_val]
for i in indexes:
index = i
most_teachers.append(teachers_list[index])
for i in most_teachers:
return i
def stats(d):
teacher = []
courses = []
list_of_lists = []
for key in d.keys():
teacher.append(key)
for value in d.values():
courses.append(len(value))
for num in range(0, len(teacher)):
list_within = [teacher[num], courses[num]]
list_of_lists.append(list_within)
return list_of_lists
2 Answers
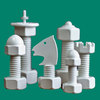
Steven Parker
231,248 PointsYou sure have a heck of a function there, but .. it worked!
But you definitely were working way to hard. Your first 3 functions were pretty concise .. I wonder why this one threw you?
But anyway, I don't normally give spoilers, but I'll show you how I did just that one:
def most_courses(d):
num = 0
for teacher in d:
if len(d[teacher]) > num:
num = len(d[teacher])
most = teacher
return most

Jon Mirow
9,864 PointsI just had a run through and came up with this (spoiler warning) https://pastebin.com/UceQr596
<<code removed to paste bin, so you don't accidentally see solution code >>
In methodology a lot of it insn't that much different, it just uses built in method calls. That makes it a bit easier to read, and they tend to be programmed in C rather than Python so run a bit faster. This is in no way a definitive list - I'm sure there are much more efficient ones, this is just a balance of what I know, what's easy to read, etc.

Jon Mirow
9,864 PointsAh I haddn't thought about people stumbling on to it by accident.. moved it to pastebin
Alex Avila
8,570 PointsAlex Avila
8,570 PointsI think I didn't know I could do something like 'most = teacher', then 'return most'. I would've guessed that would give me a key value pair from the dict. Something like '{teacher: [course1, course2, etc.]}'.
But, that is SO much simpler. Thanks!
Steven Parker
231,248 PointsSteven Parker
231,248 PointsWhen you iterate on a dictionary, you get the keys. If you want the pair you can iterate on
dict.items()
instead