Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial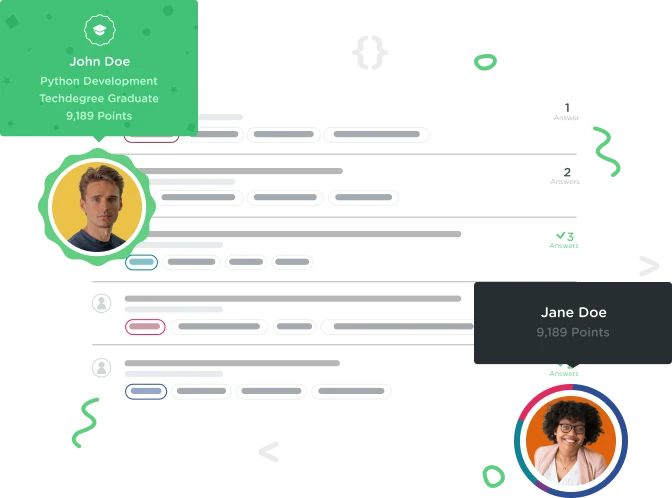

Joshua Sklodowska
1,146 PointsWhat is an argument in a function?
I did the challenge. But, I'm not sure why "count" or any particular word can be used as a function. Is there just a given list of such words for python?
If anyone can fill me in conceptually on what an argument is (the more tediously the better) I'd appreciate it. I just don't understand how
def printer count():
print("Hi " * count)
means anything in and of itself.
I feel like the video before this challenge really just showed me how to type in a function with an argument more than it explain what an argument was. For example, how would I know which argument to give a function?
I hope this wasn't too confusing a question.
Thanks!
[MOD: added ``` python markdown formatting -cf]
3 Answers
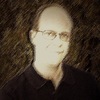
Jason Anders
Treehouse Moderator 145,860 PointsHi Joshua,
I'll see if I can explain it for you (it's been a while since I've dealt with Python).
Lets look at and break down the challenge.
def printer(count):
print("Hi " * count)
The def
key word is what is needed to "Define" a function in Python. This tells python that what follows is going to be a function.
"printer" is the name of the function being defined. This can be almost anything (within Python's namespace rules). Most languages don't allow names starting with numbers or symbols, nor can you use "Reserved" words for a given language. We could have called this function anything we wanted ("printNow", "toPrint", etc).
Now the argument. The argument goes between the parenthesis after the function name. This will be a value that is passed in when the function is called. Again, this can almost any name you want. Because of what the function does in this example, we could have called it ("printNumber", "x", "times", etc.). As long as the name of the argument chosen is used consistently throughout the function.
The print statement will print "Hi " the amount of times that was passed into "count" when the function was called. Remember, functions don't do anything until they are called. So, if we called the function and passed in 24 into the argument (named "count" here), the word "HI " (with a space) would print 24 times, and the function call would look like
printer(24)
So, basically, an argument is just another name for variable.
I hope this helps to clear some up. Keep Coding!
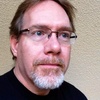
Chris Freeman
Treehouse Moderator 68,426 PointsI like Jason's answer.
I would like to clarify a finer point on the semantics. The terms "variable", "parameter", and "argument" may be used to provide a more precises discussion in code.
A "variable" is used to hold the reference to object. When you assign:
string = "some characters"
The characters "some characters
" are stored in memory and the variable string
holds the reference to that spot in memory. "string" and the address it points to is stored in the namespace of the current object or module as a key/value pair. When you id()
any variable, it is looked up in the namespace, and you get back the address of the object referred to by that variable. Variables can basically be thought of as object addresses.
When a function is defined, a list of expected parameters is defined between the parens. These parameters will receive references to objects when the function is called. That is, the parameters will become local variables with the addresses of the objects passed to the function.
When calling a function, a list of objects are provided for the function to operate on. These objects are called arguments.
So "arguments are passed to functions" and "a function can take an argument" makes more sense in this context. The phrase "define a function that takes a list" means it should expect a list
to be passed as an argument, and the function should define a parameter to receive the list.
Once this soaks in, discussing code become much clearer.
More at [Wikipedia Parameters and Arguments](https://en.wikipedia.org/wiki/Parameter_(computer_programming\)#Parameters_and_arguments)
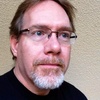
Chris Freeman
Treehouse Moderator 68,426 PointsI'll let the Monty Python masters give a Lesson in Arguments (YouTube video)