Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial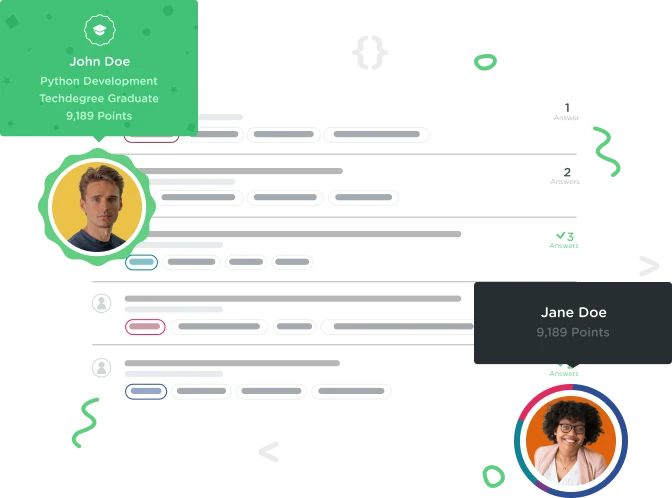
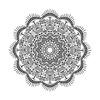
Arikaturika Tumojenko
8,897 PointsWhat is happening in this challenge? I am completely lost!
Although I managed to get the answer correct, I have no idea what's happening inside the loop. We get three random numbers for each color and I suppose we have to mix at a point. My questions are:
- how come we have 10 divs on the page and none in the html code?
- why did we multiply Math.random by 256?
-
what's the meaning of these two lines of code?
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')'; html += '<div style="background-color:' + rgbColor + '"></div>';
how can we get colors by mixing numbers?
what is happening after the counter is no longer equal to 10?
6 Answers

Erik Nuber
20,629 PointsI will try again to explain so you hopefully will understand what is going on...
Here the variables are being defined.
var html = '';
var red;
var green;
var blue;
var rgbColor;
var counter = 0;
The while loop that will start at 0 and end when counter reaches 9 for a total of ten times through the loop
while (counter <10) {
here the variable red is being set to a random number from 0 to 255. This excludes the +1 that has been used in the random number generator up to this point which means that 0 is the minimum number that can be obtained and 255 is the maximum number.
red = Math.floor(Math.random() * 256 );
the same for the green variable.
green = Math.floor(Math.random() * 256 );
and again for the blue variable
blue = Math.floor(Math.random() * 256 );
Here is where you said you were getting confused. rgbColor is storing content that looks like this...
rgb(redNum, greenNum, blueNum)
the redNum greenNum and blueNum are the numbers from 0 to 255 generated above. So the final value of rgbColor is a random rgb color ranging from rgb(0,0,0) to rgb(255,255,255)
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
Here we are taking the variable html and each time thru the loop we are adding in a div tag. The div tag will look like this
html += '<div style="background-color:' + rgbColor + '"></div>';
so html will be a series of 10 div tags and the div tag has an attribute of style which says that this particular div has a given set of CSS properties. The property being set is background-color and the value is the random rgbColor we created above.
Here we are adding to the counter which help keep the while loop going
counter +=1;
}
finally, when the loop is done, we will have a variable called html that has ten div tags each with their own style attribute giving them a background-color of a randomly generated rgbColor. and we are writing it to the screen.
document.write(html);

Erik Nuber
20,629 Pointshow come we have 10 divs on the page and none in the html code?
you are placing the div tags from the javascript file. likely there is a piece of code like
document.getElementByID("IDNameHere").innerHTML = html;
this code looks at the document, finds an ID with a specific name and, .innerHTML is used here to place the html file into this given location.
why did we multiply Math.random by 256? Colors are made up of RGB values that range from 0 to 255 so RGB values of 0,0,0 would be black up to 255, 255, 255 would be white
what's the meaning of these two lines of code?
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
html += '<div style="background-color:' + rgbColor + '"></div>';
Here you are creating RGB colors. Think of it as creating the actual CSS of an RGB color. The format for that would be
rgb(num1, num2, num3);
so the first line is setting the variable rgbColor to 'rgb(red, green, blue) the second line is adding to the variable html a div tag that has style set to a specific rgbColor that was just created in the first tag This means that the variable html is getting a div tag that has css styled to it within the div tag. when done, html will hold a total of ten div tags that will then be put onto the screen.
how can we get colors by mixing numbers?
explained above
what is happening after the counter is no longer equal to 10?
the variable html is being sent to the index.html file using the code from above.
document.getElementByID("IDNameHere").innerHTML = html;
Please note, i have not watched the video again so this is just how things should be working based on the limited info provided.

Meghana Rawate
7,144 PointsArikaturika Tumojenko
If you look at the code, the html variable can be thought of as a collector in memory as the divs are being created for the different colours. When you console log the html variable, you see that it is a long string with all of the divs next to one another. This is then put out to the browser with the document.write method (after you are out of the loop....kind of after the collection is done)
We are creating the rgbColor variable to have a different colour each time by altering the r (red), g(green), b(blue) values with the help of the loop. And each time that is done we are writing it into a div that we are storing into the html variable.
Great explanations above by everyone. I hope this helps as well.
Regards.
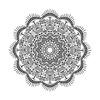
Arikaturika Tumojenko
8,897 PointsYes, actually the code is (or at least mine, since Guil used a for loop):
var html = '';
var red;
var green;
var blue;
var rgbColor;
var counter = 0;
while (counter <10) {
red = Math.floor(Math.random() * 256 );
green = Math.floor(Math.random() * 256 );
blue = Math.floor(Math.random() * 256 );
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
html += '<div style="background-color:' + rgbColor + '"></div>';
counter +=1;
}
document.write(html);
So we didn't use this code:
document.getElementByID("IDNameHere").innerHTML = html;
You explanation made thing a bit clearer, but I still have the feeling the examples used in this challenges are a bit over out head and sometimes use things we didn't cover yet. Sight.

Erik Nuber
20,629 Pointsok, yes
document.write(html);
would accomplish somewhat the same thing. this simply writes to the document.
The code I mentioned will actually place the "html" variable into a specific location with a given ID.
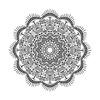
Arikaturika Tumojenko
8,897 PointsI have one more question. When exactly do we create the div? When this code runs?
html += '<div style="background-color:' + rgbColor + '"></div>';
So we actually generate a div which is already styled and we keep adding one until the loops runs 10 times, after the code exits the loop and we see the whole 10 divs at once?

Erik Nuber
20,629 PointsYes exactly by the end of the while loop you will have this
html = '<div style="background-color:' + rgbColor + '"></div>' + '<div style="background-color:' + rgbColor + '"></div>' + '<div style="background-color:' + rgbColor + '"></div>' + '<div style="background-color:' + rgbColor + '"></div>' + '<div style="background-color:' + rgbColor + '"></div>' + '<div style="background-color:' + rgbColor + '"></div>' + '<div style="background-color:' + rgbColor + '"></div>' + '<div style="background-color:' + rgbColor + '"></div>' + '<div style="background-color:' + rgbColor + '"></div>' + '<div style="background-color:' + rgbColor + '"></div>';
by using the += it just cuts down on the messiness and, we aren't storing each of these div tags in individual variables.

Meghana Rawate
7,144 PointsOh..In your code you have used the random method to create the RGB values. So you are just using the loop to create a certain number of divs (ie dots of colour)
Arikaturika Tumojenko
8,897 PointsArikaturika Tumojenko
8,897 PointsYes, thank you so much. I understand exactly how the code works but I am stuck at this part of the code
html += '<div style="background-color:' + rgbColor + '"></div>';
This piece, more specific (as I know the syntax for the <div style="background-color: rgb(a, b, c)"></div>
"background-color:' + rgbColor + '"
What do we concatenate here? Background-color goes between a pair of " ". So what's the meaning of ' + rgbColor + '? Sorry for asking so many questions, but I really don't get this syntax. Maybe I'm just tired and I don't see the obvious (I pair the quotes incorrectly).
Erik Nuber
20,629 PointsErik Nuber
20,629 Pointshere is what we are going for
<tagname style="property:value;">
'<div style="background-color:' + rgbColor + '"></div>';
The quotes are needed around the property: value pair. If anything what is missing is a semi-colon. I mean it is the single quotes you should be looking at here. The double quotes are needed around the property: value pair.
Here is the first part.
'<div style="background-color:'
here is the second part
+ rgbColor +
and the final part
'"></div>';