Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial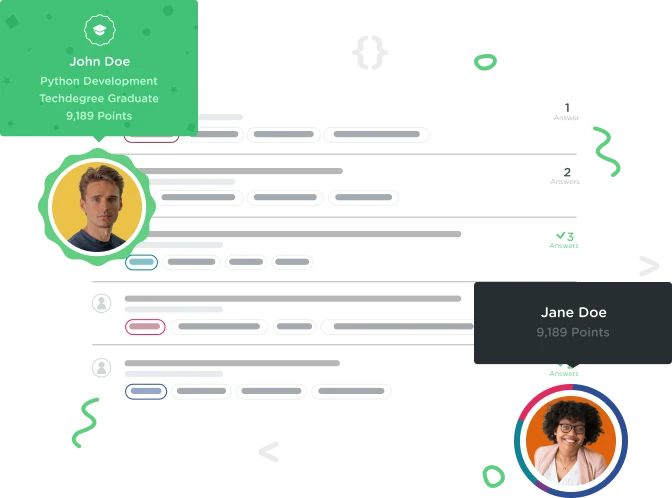

Adam Laszlo Vincze
4,083 PointsWhat is problem with my program?
var RightAnswer = 0;
var name = prompt("Please tell me your name");
alert("Click ok and quiz will be starting!");
var ques = prompt("How much is 2^3*3^3?");
var ans = parseInt(ques);
if (ans === 216 ) {
alert("It's correct");
}
RightAnswer = RightAnswer+1;
else {
alert("It's wrong. Right is 216");
}
var ques2 = prompt("How much is 2*8?");
var ans2 = parseInt(ques2);
if (ans2 !== 16 ) {
alert("It's wrong. Right is 16");
}
if else (ans2 === 16){
alert("It's correct.");
}
RightAnswer = RightAnswer+1;
else {
alert("It's wrong. Right is 16")
}
var ques3 = prompt("How much is 256^0?");
var ans3 = parseInt(ques3);
if (ans3 === 1 ) {
alert("It's correct");
}
RightAnswer = RightAnswer+1;
if else (ans3 < 1 || ans3 >1 ) {
alert("It's wrong. Right is 1");
}
else{
alert("It's wrong. Right is 1");
}
var ques4 = prompt("How much is 7+8*12^0?");
var ans4 = parseInt(ques4);
if (ans4 === 15 ) {
alert("It's correct");
}
RightAnswer = RightAnswer+1;
else {
alert("It's wrong. Right is 15");
}
var ques5 = prompt("How much is 6+8?");
var ans5 = parseInt(ques5);
if (ans5 === 14 ) {
alert("It's correct");
}
RightAnswer = RightAnswer+1;
if else (ans5 <14 && ans5 !==14) {
alert("It's wrong. Right is 14");
}
else {
alert("It's wrong. Right is 14");
}
var ques6 = prompt("How much is 2+(-1)?");
var ans6 = parseInt(ques6);
if (ans6 === 1 ) {
alert("It's correct");
}
RightAnswer = RightAnswer+1;
else {
alert("It's wrong. Right is 216");
}
if (RightAnswer === 6) {
alert("Congratulations" + " " + name + " " + "you have" + " " + RightAnswer + " " + "right answer so you earn a gold medal!")
}
if else (RightAnswer >=3 && RightAnswer < 6) {
alert("Congratulations" + " " + name + " " + "you have" + " " + RightAnswer + " " + "right answer so you earn a silver medal!")
}
if else (RightAnswer >=1 && RightAnswer <3) {
alert("Congratulations" + " " + name + " " + "you have" + " " + RightAnswer + " " + "right answer so you earn a bronze medal!")
}
else {
alert("Please try again!")
}
3 Answers
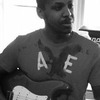
Samuel Webb
25,370 PointsLike Jasper said, your if/else if/else statements were messed up. You want to make sure RightAnswer is inside of the statement so that it will all run before the next statement. There were 2 problems with the way you had it. Firstly, you can't put a statement between the if and the else statements because after it runs that middle statement, it finds a lone else statement which is required to come after an if or else if statement. So this won't work:
if(and === 14) {
alert("It's correct");
}
RightAnswer = RightAnswer + 1;
else {
alert("Wrong");
}
The other issue with that previous code block is that RightAnswer will be added to no matter if it's right or wrong since it's outside of the if block.
Another issue I encountered was that you were saying "if else" instead of "else if". In the last set of if/else if/else statements, you forgot to put the semicolon after the alerts. That's pretty much everything I can remember. I've corrected everything and here's the working code.
var RightAnswer = 0;
var name = prompt("Please tell me your name");
alert("Click ok and quiz will be starting!");
var ques = prompt("How much is 2^3*3^3?");
var ans = parseInt(ques);
if (ans === 216 ) {
alert("It's correct");
RightAnswer = RightAnswer + 1;
} else {
alert("It's wrong. Right is 216");
}
var ques2 = prompt("How much is 2*8?");
var ans2 = parseInt(ques2);
if (ans2 !== 16 ) {
alert("It's wrong. Right is 16");
} else {
alert("It's correct.");
RightAnswer = RightAnswer + 1;
}
var ques3 = prompt("How much is 256^0?");
var ans3 = parseInt(ques3);
if (ans3 === 1 ) {
alert("It's correct");
RightAnswer = RightAnswer + 1;
} else {
alert("It's wrong. Right is 1");
}
var ques4 = prompt("How much is 7+8*12^0?");
var ans4 = parseInt(ques4);
if (ans4 === 15 ) {
alert("It's correct");
RightAnswer = RightAnswer + 1;
}
else {
alert("It's wrong. Right is 15");
}
var ques5 = prompt("How much is 6+8?");
var ans5 = parseInt(ques5);
if (ans5 === 14 ) {
alert("It's correct");
RightAnswer = RightAnswer + 1;
} else {
alert("It's wrong. Right is 14");
}
var ques6 = prompt("How much is 2+(-1)?");
var ans6 = parseInt(ques6);
if (ans6 === 1 ) {
alert("It's correct");
RightAnswer = RightAnswer + 1;
} else {
alert("It's wrong. Right is 216");
}
if (RightAnswer === 6) {
alert("Congratulations" + " " + name + " " + "you have" + " " + RightAnswer + " " + "right answer so you earn a gold medal!");
} else if (RightAnswer >= 3 && RightAnswer < 6) {
alert("Congratulations" + " " + name + " " + "you have" + " " + RightAnswer + " " + "right answer so you earn a silver medal!");
} else if (RightAnswer >=1 && RightAnswer <3) {
alert("Congratulations" + " " + name + " " + "you have" + " " + RightAnswer + " " + "right answer so you earn a bronze medal!");
} else {
alert("Please try again!");
}
Sorry it's so indented, that's how it was when I was working with it.
I would also suggest using a while loop so that the questions can be restarted at the end instead of the person having to refresh the page. Cheers mate, hope this helps.
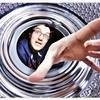
Jasper Leenarts
13,830 PointsFor starter, you have some else if statements wrong. For example:
var ques5 = prompt("How much is 6+8?");
var ans5 = parseInt(ques5);
if (ans5 === 14 ) {
alert("It's correct");
}
RightAnswer = RightAnswer+1;
if else (ans5 <14 && ans5 !==14) {
alert("It's wrong. Right is 14");
}
else {
alert("It's wrong. Right is 14");
}
should be more something like this:
var ques5 = prompt("How much is 6+8?");
var ans5 = parseInt(ques5);
if (ans5 === 14 ) {
alert("It's correct");
}
RightAnswer = RightAnswer+1;
else {
alert("It's wrong. Right is 14");
}
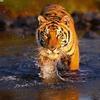
Konrad Pilch
2,435 PointsOh wow :D somebody replied. it seemed long to me but woahhh , people actually read the code .
Thats awesome! ^^
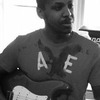
Samuel Webb
25,370 PointsAdding to RightAnswer should actually be done inside the if statement like this:
var ques5 = prompt("How much is 6+8?");
var ans5 = parseInt(ques5);
if (ans5 === 14 ) {
alert("It's correct");
RightAnswer = RightAnswer + 1;
} else {
alert("It's wrong. Right is 14");
}

Adam Laszlo Vincze
4,083 PointsThanks For help samuel and everybody :)
Samuel: Dave Mcfarland didn't teach loops yet in the videos ;)
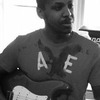
Samuel Webb
25,370 PointsOh ok. Well keep this project saved somewhere and when you learn loops, you should try to add one to make it repeatable. Something to think about is keeping a lot of your projects from when you start coding. Then when you've learned a lot more, go back to those old projects and see how much better you can make them. It's a great way to use the new skills you learn.
Konrad Pilch
2,435 PointsKonrad Pilch
2,435 PointsHi, i suggest you that you download the code and you can compare them . Thats what i do most of the times.