Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial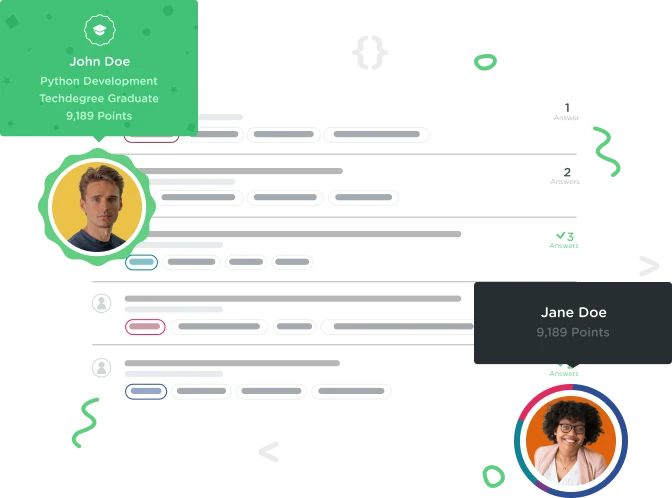

tommy huynh
Full Stack JavaScript Techdegree Student 3,723 PointsWhat is returned when splitting a string?
When splitting a string with ".split", how is that string returned? Is it in an array form or separate strings?
9 Answers
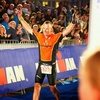
Steve Hunter
57,712 PointsHi Tommy,
Try using inspect
on the returned value to test this assertion; but I think it returns an array of sub-strings.
Steve.

tommy huynh
Full Stack JavaScript Techdegree Student 3,723 PointsHey Steve,
What does .inspect do?
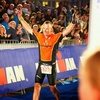
Steve Hunter
57,712 PointsHi Tommy,
I can't remember if inspect
is an irb thing only or if it works in standard Ruby. But it pulls back all the details of the instance you send it. So, it'll tell the data type, i.e. String array, Dictionary etc.
I'm not at a machine where I can test that right now, unfortunately. I'll try tomorrow; 23:15 here now.
Steve.

tommy huynh
Full Stack JavaScript Techdegree Student 3,723 PointsThanks a lot Steve!
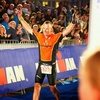
Steve Hunter
57,712 PointsReally not a problem! By way of understanding; why the question relating to split()
?

tommy huynh
Full Stack JavaScript Techdegree Student 3,723 PointsIt is for a coding challenge where I have to find the longest word in a string. I'm super new to coding so some of the basic stuff doesn't make sense to me yet but I'm getting there slowly.
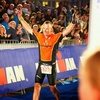
Steve Hunter
57,712 PointsInteresting challenge!
I just ran a IRB session and can confirm that split
returns an array of strings. [irb](tryruby.org) is great!
So, how do you want to iterate over those array elements? How to test their length?
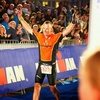
Steve Hunter
57,712 PointsOK - I've got some code that does the job.
What do you understand, what makes no sense and how would you approach the challenge?
Happy to walk you through this. And, by the way; why Ruby?! Personally, I love the language but that doesn't make it the best choice. But hey, that's what we've got to work with, so let's GO!

tommy huynh
Full Stack JavaScript Techdegree Student 3,723 PointsHey Steve,
The code challenges are to prepare me for the application process to get admitted into a Coding Bootcamp. I will be away from my computer for a bit but once I get back on I'll refer back to the problem and let you know where I'm stuck and what my thought process for the challenge is!
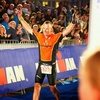
Steve Hunter
57,712 PointsHappy to help talk through your thoughts and a solution. As I say, I just whipped up some working code for this one.

tommy huynh
Full Stack JavaScript Techdegree Student 3,723 PointsWrite a method that takes in a string. Return the longest word in the string. You may assume that the string contains only letters and spaces.
I can look up the answer but I really want to solve this problem myself.

tommy huynh
Full Stack JavaScript Techdegree Student 3,723 PointsI'm stuck. Here's my code and I do not know why it's not working.
def longest_word(sentence)
words = sentence.split(" ")
longest_word = 0
i = 0
while i < words.length
longest_word = words[i].length
if words[i].length > longest_word
longest_word = words[i].length
end
i += 1
end
return longest_word
end
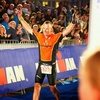
Steve Hunter
57,712 PointsHi Tommy,
Apologies for the delay; I needed some sleep and then had training to do this morning.
Right, on to your method. First, the challenge says to return longest word. What you're returning is the length of the longest word. That right?
Steve.
(I'm just typing your code so I'll test your method shortly - I can see one issue already)
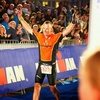
Steve Hunter
57,712 PointsOK - so this method returns the length of the last word in the sentence. Why?
Here:
while i < words.length
longest_word = words[i].length
At every iteration of the loop, the length of the current word in the loop is assigned to longest_word
. That line needs omitting as it is repeated, correctly, in the if
statement. I'll comment it out and see what we get then.
That now returns the length of the longest word but I'm not sure that's what the test is wanting it to do.
If you want it to return the actual word, you just need to add a couple of lines to your code. Create a variable at the top of the method to hold a string; initialize it to be an empty string. Then, inside your if
statement, assign words[i]
into it. Return that variable at the end of the method.
Also, experiment with an each
loop. It is nicer syntax than this while
loop. Something like:
words = sentence.split()
words.each do |word|
# do some to each word
end
This will hold each word in the sentence in the local variable (which can be called anything) which I called word
. So you can put your amended if
conditional inside that and it'll do the same job without needing to test the length of the array or increment a counter variable.
Make sense?
Steve.

tommy huynh
Full Stack JavaScript Techdegree Student 3,723 PointsI was able to solve it.
def longest_word(sentence)
words = sentence.split(" ")
longest_word = 0
i = 0
while i < words.length
current_word = words[i]
if longest_word == 0
longest_word = current_word
elsif longest_word.length < current_word.length
longest_word = current_word
end
i += 1
end
return longest_word
end
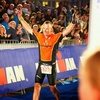
Steve Hunter
57,712 PointsWell done. A couple of points.
When you've just set longest_word
to be equal to zero, following that with a test to see if longest_word
is equal to zero is writing code that doesn't need to be there!
You just want to go straight into your comparison of longest_word.length < current_word.length
which is what does the real work!
Although, longest_word
starts out as being zero - an integer. It then becomes current_word
which is a string. You can also trim your code still further by not using current_word
at all. Just use words[i]
they're the same thing. That gets rid of a little duplication.
And, genuinely, consider using an .each
loop. It is the most common Ruby loop construct and way easier to manage as there's no [i]
stuff and incrementing i
. It just works way more easily.
I'm not at a compiler right now but this could be something like:
def longest_word(sentence)
words = sentence.split() # defaults to space delimiter
# can we loop through sentence.split() without needing words variable? Check!
longest_word = "" # this isn't a number!
words.each do | word |
if word.length > longest_word.length
longest_word = word
end
end
longest_word # don't need the return keyword in Ruby
end
I'm pretty sure we could use sentence.split().each do | word |
to remove a line or two but can't check that now.
I'll try that tomorrow to see if it works OK but do you see how easier that is from the while
structure? When you have an object capable of being iterated over, to use Python parlance; 'an iterable'; you can use each
to divide it up and loop really quickly and easily over each element.
I hope that helps - assuming it works! I'm on my tablet downstairs, the work machines are doing updates & backups in the office upstairs.
Steve.