Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial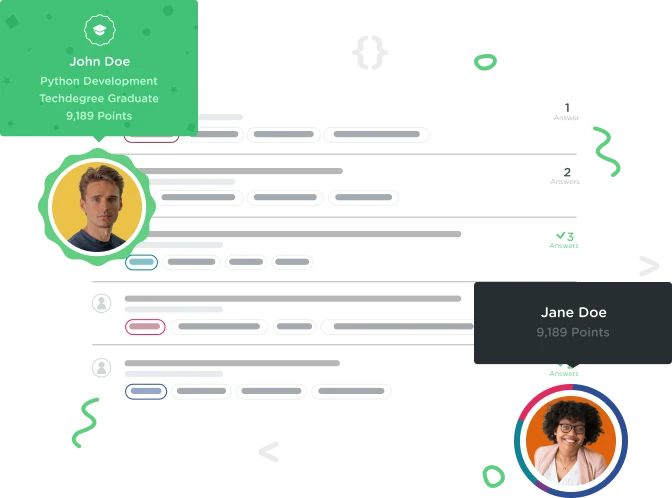
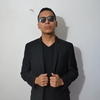
Cristian Castro
Courses Plus Student 12,666 PointsWhat is the difference between [] and () in this code challenge?
I was solving this code challenge and I came across this... var temperatures = [100,90,99,80,70,65,30,10]; for ( var i = 0; i < temperatures.length; i += 1) { console.log(temperatures(i)) } It said it was wrong. Then I did this... var temperatures = [100,90,99,80,70,65,30,10]; for ( var i = 0; i < temperatures.length; i += 1) { console.log(temperatures[i]) } and it was right.
My question is... What happens if I use ()?
var temperatures = [100,90,99,80,70,65,30,10];
for ( var i = 0; i < temperatures.length; i += 1) {
console.log(temperatures[i])
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
3 Answers

Joey Ward
Courses Plus Student 24,778 PointsWhen you use "temperatures(i)", the browser thinks you are passing the value of a variable "i" as an argument to a function "temperatures".
If "temperatures" is an array (not a function) the. The browser won't know what to do when your code is written as if it's trying to pass an argument to a function.
To call an index from an array, you would use [] rather than ().
Since your code is looping over an array and calling the value at each index, you want to use "temperatures[i]".

Kevin Delva
1,543 PointsFrom what I understand 'temperature' is the array, and to access any item in an array you would use it like this: temperature[x], x being the index in which the item in the array is. So in this case: console.log(temperature[i]); i is the variable that is representing the index in which the item of the array is (and it will be changing according to your for loop), so just as you would access an item in an array like this temperature[x], you can't use "( )" in the code, as only brackets "[ ]" can be used for it. I'm still quite new, sorry if I couldn't explain it better for you. Hope I could help! :)
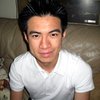
jason chan
31,009 Pointsvar temperatures = [100,90,99,80,70,65,30,10];
temperatures = temperatures.reverse();
for ( var i = 0; i < temperatures.length; i += 1) {
console.log(temperatures[i])
}
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/reverse
That's how i did it. I used reverse.