Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial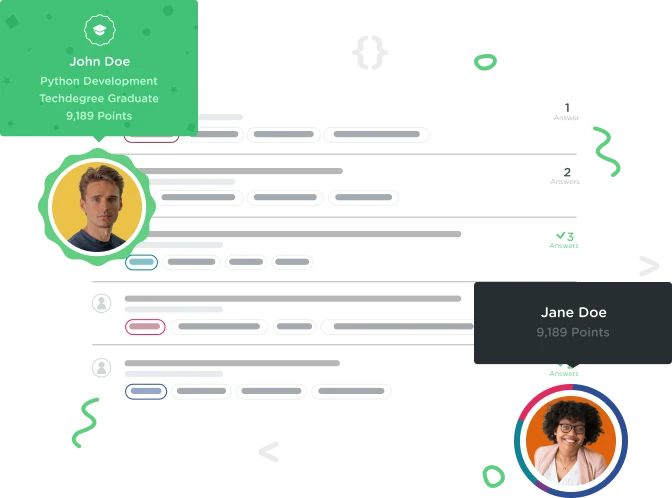
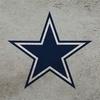
Anmol Gulati
13,991 PointsWhat is the error in my code?
I've run the same code in tree house work space and it is running fine
var count ='';
for ( var i= 4; i <=156 ; i += 1)
{
count += i;
}
console.log(count);
2 Answers

Thomas Fildes
22,687 PointsHi Amnol,
The correct code is below:
for (i = 4; i <= 156; i++) {
console.log(i);
}
In the above code, the i++ increments i by 1 every time the i <= than 156. you don't need to use a separate variable to log this. The console.log will run every time the condition is fulfilled with i as the output.
Hope this helps! Happy Coding!!!
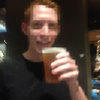
Sebastian H
19,905 PointsHey Anmol,
Your error is in your variable declaration at the top of your code:
var count = '';
In this line you are setting a variable to be a string type, but you are then trying to add numbers to it. This results in an error or unexpected result. To solve this problem, simply define your 'count' variable as a number to start with :)
ex.
var count = 0;
I'm not sure I explained that particularly well so here is a short quote from this page which explains javascript data types and the type of problem you have run into:
The variable’s data type is the JavaScript scripting engine’s interpretation of the type of data that variable is currently holding. A string variable holds a string; a number variable holds a number value, and so on. However, unlike many other languages, in JavaScript, the same variable can hold different types of data, all within the same application. This is a concept known by the terms loose typing and dynamic typing, both of which mean that a JavaScript variable can hold different data types at different times depending on context.
With a loosely typed language, you don’t have to declare ahead of time that a variable will be a string or a number or a boolean, as the data type is actually determined while the application is being processed. If you start out with a string variable and then want to use it as a number, that’s perfectly fine, as long as the string actually contains something that resembles a number and not something such as an email address. If you later want to treat it as a string again, that’s fine, too.
The forgiving nature of loose typing can end up generating problems. If you try to add two numbers together, but the JavaScript engine interprets the variable holding one of them as a string data type, you end up with an odd string, rather than the sum you were expecting. Context is everything when it comes to variables and data types with JavaScript.