Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial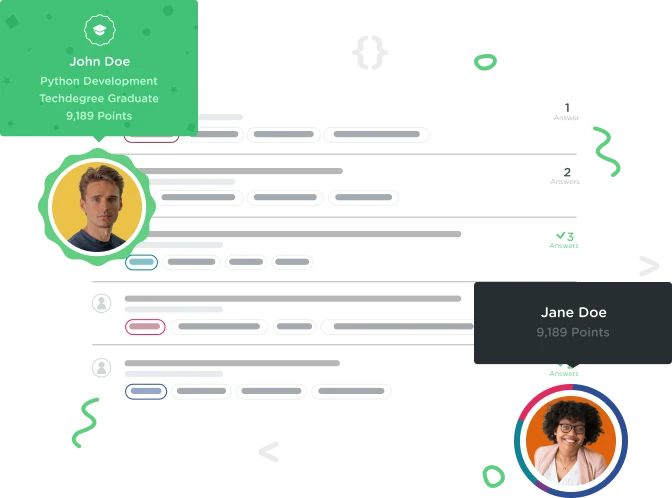

Gary Gibson
5,011 PointsWhat is the .item() doing?
https://teamtreehouse.com/library/python-collections/dictionaries/teacher-stats
The question is: "...create a function name stats that takes the teacher dictionary. Return a list of lists in the format [<teacher name>, <number of classes>].
def stats(dict):
a_list = []
for teacher, class_num in dict.items():
new_string = [teacher, len(class_num)]
a_list.append(new_string)
return a_list
The above code works. But I'm not clear on why the .items() is there. Please help.
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
def most_classes(dict):
most_teach = ""
current_count = 0
for teacher in dict:
if len(dict[teacher]) > current_count:
current_count = len(dict[teacher])
most_teach = teacher
return most_teach
1 Answer
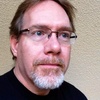
Chris Freeman
Treehouse Moderator 68,460 PointsWhen a dictionary is used in an iterator context such as if a for
loop, the default action is to iterate over the keys of the dict.
# these are equivalent statements
if teacher in teachers:
if teacher in teachers.keys()
There are two other ways to iterate a dictionary, by the *values using the .values()
method, and by iterating over *both keys and values using the .items()
method.
The .items()
method will return two objects: a key and its corresponding value. This is why the for
loop as two loop variables. The first receives the key, the second receives the value.
So instead of getting just the key then looking up the value, by using items()
you can get the both at the same time which can be faster and less coding.
Gary Gibson
5,011 PointsGary Gibson
5,011 PointsThank you. It looks like this is something a more advanced student came up with. I thought I was supposed to have been able to use it.