Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial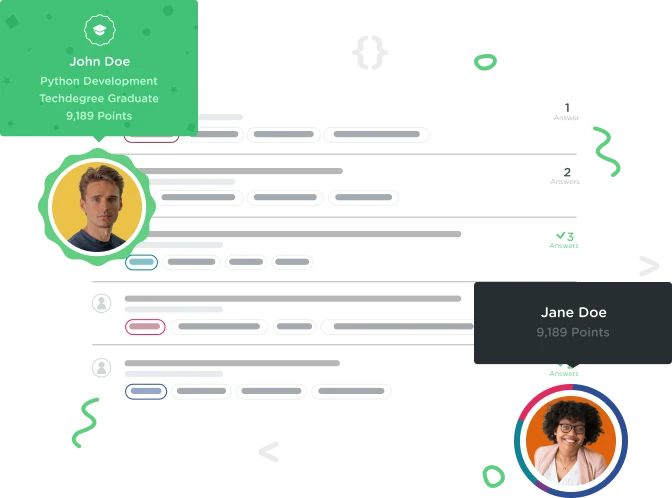

Jonathan Shedd
1,921 PointsWhat is the "return not num % 2" statement supposed to do in this code?
I feel like I've written the code correctly but it keeps saying that there are not enough prints. Also, I'm not entirely sure why the "return not num % 2" statement was added at the end. Any help is greatly appreciated!
import random
start = 5
def even_odd(num):
while start == True:
num = random.randint(1, 99)
if num % 2 == 0:
print("{} is even".format(num))
else:
print("{} is odd".format(num))
start = start - 1
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
2 Answers

Robin *
5,670 PointsYep, place while loop under the odd_even function (not in it) then use the func to check if the random number is even if not its odd. Also while start: is that same as while start == True so u can drop that bit. I've written my solution under so if you don't want spoilers don't look :D
import random
start = 5
def even_odd(num):
return not num % 2
while start:
num = random.randint(1, 99)
if even_odd(num):
print('{} is even'.format(num))
else:
print('{} is odd'.format(num))
start -= 1
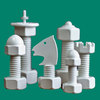
Steven Parker
231,269 Points
"return not num % 2
" is the body of the even_odd function.
You should not modify this provided function. All of your new code should be outside of this function.
In task 3, you will call this function inside your new loop to check the chosen number.
Jonathan Shedd
1,921 PointsJonathan Shedd
1,921 PointsThanks for the help! I was finally able to complete the challenge without looking at the code that you wrote for the challenge. :)