Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial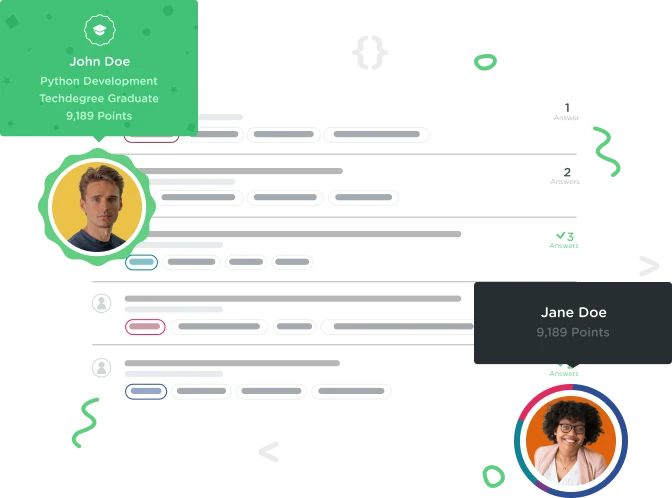

sonam rathore
703 Pointswhat is the solution of this question?
how can the round function used for uppercase and lowercase of string?
# The first half of the string, rounded with round(), should be lowercased.
# The second half should be uppercased.
# E.g. "Treehouse" should come back as "treeHOUSE"
4 Answers

Script Code
6,631 PointsI think you misinterpreted the challenge. The challenge is create a function that takes a string return a string with first half in uppercase and second half in lowercase. You are not using the round function to process strings. First thing, you will need to split the string down in half. The reason you are asked to use round is that len(string) could return an even or uneven number. An uneven number would return a float (e.g 5/2=2.5). As you cannot index using a float you use round for the challenge.
round(5/2) # returns 2
So for for a string with 5 characters, the first 2 characters (index 0, 1) are considered your first half and the remaining 3 characters the second half.
def sillycase(myString):
length = len(myString)
split = round(length/2)
first_half = myString[:split].lower() # notice the position of the colon
second_half = myString[split:].upper() # again, notice the position of the colon
return first_half + second_half
Let me know if you need more help.

Chris Jones
Java Web Development Techdegree Graduate 23,933 PointsHey sonam,
I used the round function to find the halfway point (rounded to an integer or whole number) of the string. If the string that is passed into the function's parameter has an even number of characters, you won't need to use the round function, but if the string has an odd number of characters, you will need it so it's good to have it in the function's logic. Here's what I did in case you need a reference.
def sillycase(string):
halfwayPoint = round(len(string)/2) # get halfway point of function's string argument.
firstHalf = string[:halfwayPoint].lower() # convert first half of string parameter to lowercase.
secondHalf = string[halfwayPoint:].upper() # convert second half of string parameter to uppercase.
return firstHalf + secondHalf

sonam rathore
703 Pointsthanks chris jones.

Chris Jones
Java Web Development Techdegree Graduate 23,933 PointsNot a problem! I'm glad it helped! Keep it up!

sonam rathore
703 Pointsthanks for such a good explaination for round function in strings.