Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial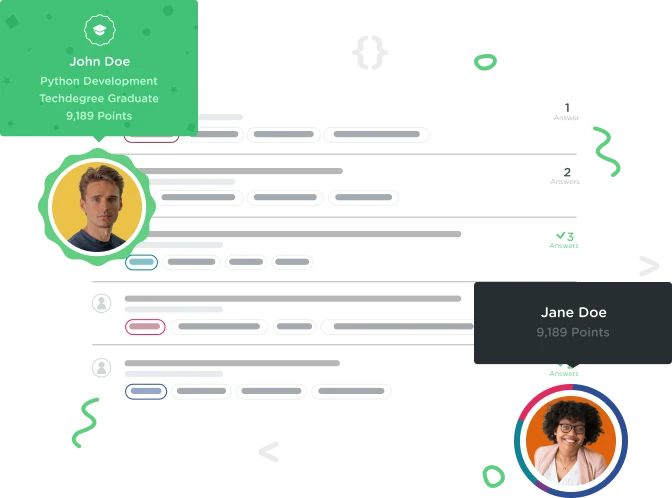

George Lugo
Python Web Development Techdegree Student 922 Pointswhat is wrong with my code
def sillycase(string): return sillycase.lower[:5] + sillycase.upper[5:]
def sillycase(string):
return sillycase.lower[:5] + sillycase.upper[5:]
6 Answers
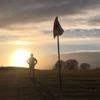
Stuart Wright
41,119 Points.upper() and .lower() are both methods, which means they require parentheses on the end of each (but before your square brackets start).
Also note that you should call these on the string variable (which you have called 'string'), rather than on the function name as you have.
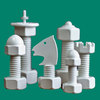
Steven Parker
230,995 PointsFYI: These ( )
are parentheses.
And these { }
are curly brackets.
Methods use parentheses.
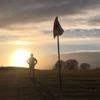
Stuart Wright
41,119 PointsAh yes of course, thanks - will edit my post.
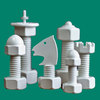
Steven Parker
230,995 PointsHere's a few hints:
- when you call a method, you must have parentheses after the method name (even if it takes no arguments)
- your return value should be made using the argument ("string")
- the function should work with arguments of any length (not just 9 or 10)
- recursion is not needed here, so the function should not refer to itself

George Lugo
Python Web Development Techdegree Student 922 Pointsthe only problem is understand i have to do it with arguments of any length. but how do i cut it in half to return the value of these arguments.
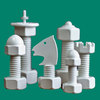
Steven Parker
230,995 PointsYou might do something like this to get an integer approximately half the size:
halfsize = int( len(mystring) / 2 )

George Lugo
Python Web Development Techdegree Student 922 Pointsyes but how would you do both upper and lower would it be two different variables like shown
half size = int(len(mystring)/2) half size2 = int(len(mystring)/2)
return half size.lower()
return half size2.upper()
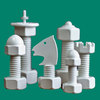
Steven Parker
230,995 PointsYou might plug that value into your slices. In both cases it would replace where you had the fixed value "5" before.
Also, note that variable names cannot have spaces in them.

George Lugo
Python Web Development Techdegree Student 922 Pointsdef sillycase(mystring): string = (int(len(mystring / 2)) string2 = (int(len(mystring / 2)) return string.lower() + string2.upper()

George Lugo
Python Web Development Techdegree Student 922 Pointsdef sillycase(mystring): return mystring.lower[(int(len(mystring / 2)))] + mystring.upper[(int(len(mystring / 2)))] i tried this and it is still not working
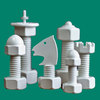
Steven Parker
230,995 PointsYou're missing some of what you started with, and you're not using all the hints you have above.
You have some new errors that look like something done in a hurry. Don't be too hasty.
Steven Miyakawa
14,540 PointsSteven Miyakawa
14,540 PointsInside your sillycase function, 'string' is the variable that will hold the value of the string passed in. So you can call methods such as 'lower' and 'upper' on 'string'. You can also slice the variable 'string'.
Example of slicing a string:
Example of calling lower on a string:
The sillystring problem has three parts. First, you need to split the string passed in ('string' in your case) into two strings split down the middle (hint: you can use slicing to do this). For example, 'asdf' should become 'as' and 'df'. Next, you have to convert the first half to lowercase and the second half to uppercase (hint: you can use the 'lower' and 'upper' methods to do this). Lastly, you need to concatenate the first half and the second half and return that value.