Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial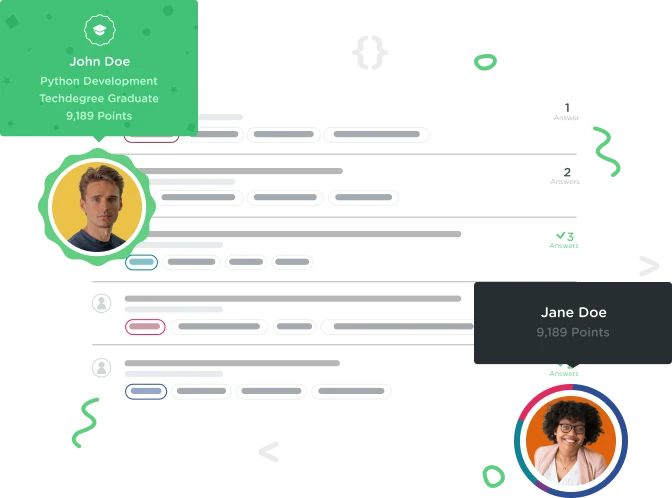

michael singh
5,927 PointsWhat is wrong with my code?
var students = [
{
name: 'mike',
track: 'Front End Development',
acheivements: 15,
points: 5100,
},
{
name: 'nicki',
track: 'Design',
acheivements: 12,
points: 1800,
},
{
name: 'Megan',
track: 'Back End Development',
acheivements: 23,
points: 7691,
},
{
name: 'nathan',
track: 'Software Dev',
acheivements: 7,
points: 900,
},
{
name: 'kyle',
track: 'Design',
acheivements: 5,
points: 2300,
}
];
var search;
var student;
var message = '';
function print(message) {
var outputDiv = document.getElementById("output");
outputDiv.innerHTML = message;
}
function getStudentReport(student){
var report = '<h2><strong>Student: ' + student.name + '</strong></h2>';
report += '<h2>Track: ' + student.track + '</h2>';
report += '<h2>Acheivements: ' + student.acheivements + '</h2>';
report += '<h2>Points: ' + student.points + '</h2>';
return report;
}
while(true){
search = prompt('Search for a student. Enter quit to exit');
if(search === null || search.toLowerCase() === 'quit'){
break;
}
for(var i = 0; i < students.length; i += 1){
student = students[i];
if(student.name.toLowerCase() === search.toLowerCase()){
message = getStudentReport(student);
print(message);
}
if(student.name.toLowerCase() !== search.toLowerCase()){
message = '<h2>Sorry that student does\'t exist</h2>';
print(message);
}
}
}
(
1 Answer

Seth Kroger
56,413 PointsWhat your for loop does is change the message every time through the loop. The problem is when you find the student you're looking for the loop will continue on and erase the results on the next iteration, because the student you're searching for won't the be equal to the new student (unless you happen to be searching for the last student in the array.
Perhaps a better approach is to set the not-found message first and replace it with the results when the desired student is found. Then you can print the message out when you're done.
message = '<h2>Sorry that student does\'t exist</h2>';
for(var i = 0; i < students.length; i += 1){
student = students[i];
if(student.name.toLowerCase() === search.toLowerCase()){
message = getStudentReport(student);
break;
}
}
print(message);
Also the points: xxx lines shouldn't have commas at the end, since they're the last property of the object.