Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial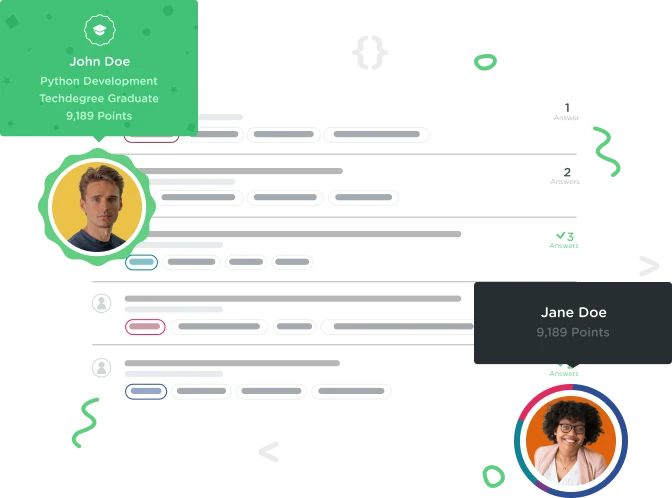

Małgorzata Staniszewska
2,431 Pointswhat is wrong with my code?
?
def add(a,b):
try:
except ValueError:
return(None)
else:
return(float(a)+float(b))
1 Answer

andren
28,558 PointsYou have the structure of try statements slightly off, a try statement is structured like this:
try:
#CODE TO BE TRIED
except #ERROR TYPE:
#CODE TO BE RUN IF ERROR OCCURS
else:
#CODE TO RUN IF ERROR DOES NOT OCCUR
# It is important to note that try, except and else all need to be on the same indentation level.
In your code you are not actually providing any code to be tried, and you have also placed the except and else statement within the try block indentation wise which is incorrect.
The solution to this task looks like this:
def add(a,b):
try:
float(a)
float(b)
except ValueError:
return(None)
else:
return(float(a)+float(b))
In the above code I don't to anything useful inside the try block, I just convert a and b to floats without actually storing the result of that conversion anywhere, but that is fine since the point of the try clause in this instance is just to see if a and b can be converted to floats without causing any errors. If they can be then the code within the else clause is executed, if they can't then the code within the except clause is executed.
Rustam Ismailov
6,234 PointsRustam Ismailov
6,234 PointsTry to use this format :