Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial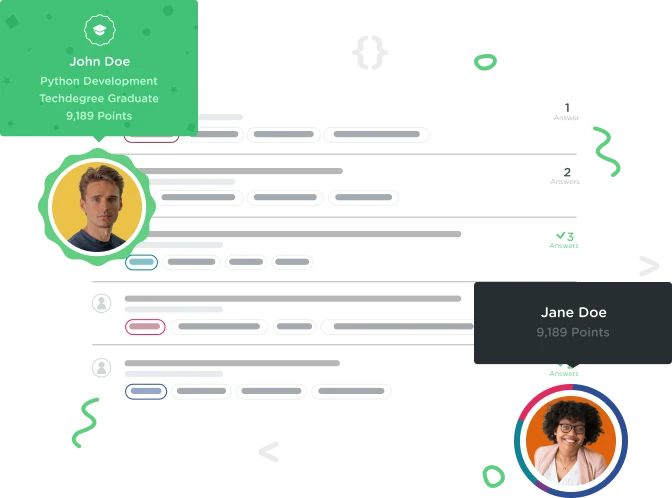

Razvan Sighinas
2,018 PointsWhat is wrong?Please help me
import android.app.Activity;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import java.util.Random;
public class funfacts2 extends Activity {
private FactBook mFactBook = new FactBook();
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_funfacts2);
// Declare our View variables and assign them the views from the Layout file
final TextView factLabel = (TextView) findViewById(R.id.factTextView);
Button showFactButton = (Button) findViewById(R.id.showFactButton);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View view) {
String fact = mFactBook.getFact();
//update the label with our dynamic fact
factLabel.setText(fact);
}
};
showFactButton.setOnClickListener(listener);
}
1 Answer
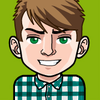
Harry James
14,780 PointsHey Razvan!
It looks like you've got the curly brackets in your code a bit mixed up. I've gone ahead and formatted it for you so that it's easier to see where you've gone wrong and also put comments where the start and end brackets are:
import android.app.Activity;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import java.util.Random;
public class funfacts2 extends Activity { // Start bracket for class
private FactBook mFactBook = new FactBook();
@Override
protected void onCreate(Bundle savedInstanceState) { // Start bracket for onCreate()
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_funfacts2);
// Declare our View variables and assign them the views from the Layout file
final TextView factLabel = (TextView) findViewById(R.id.factTextView);
Button showFactButton = (Button) findViewById(R.id.showFactButton);
View.OnClickListener listener = new View.OnClickListener() { // Start bracket for anonymous inner class of OnClickListener()
@Override
public void onClick(View view) { // Start bracket for onClick() method
String fact = mFactBook.getFact();
//update the label with our dynamic fact
factLabel.setText(fact);
} // End bracket for onClick() method.
}; // End bracket for anonymous inner class of OnClickListener()
showFactButton.setOnClickListener(listener);
} // End bracket for onCreate()
} // End bracket for class
Go ahead and paste this code into Android Studio, it should then be formatted in there which is easier to see than it is on here:
If we look at your code again:
public class funfacts2 extends Activity { // Start curly bracket
private FactBook mFactBook = new FactBook();
} // End curly bracket
You're actually ending your class after declaring the FactBook variable - which isn't what you want.
Hopefully this should explain where you've gone wrong but if there's something you don't quite understand, give me a shout and I'd be happy to further explain it for you :)
P.S: Here's a version without the comments:
import android.app.Activity;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import java.util.Random;
public class funfacts2 extends Activity {
private FactBook mFactBook = new FactBook();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_funfacts2);
// Declare our View variables and assign them the views from the Layout file
final TextView factLabel = (TextView) findViewById(R.id.factTextView);
Button showFactButton = (Button) findViewById(R.id.showFactButton);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View view) {
String fact = mFactBook.getFact();
//update the label with our dynamic fact
factLabel.setText(fact);
}
};
showFactButton.setOnClickListener(listener);
}
}
Caleb Kleveter
Treehouse Moderator 37,862 PointsCaleb Kleveter
Treehouse Moderator 37,862 PointsHey Razvan,
Can you post the instructions for the challenge? Also, it makes it easier to read the code if you post in markdown, like below.
The bullet points are there so you can see how to write the markdown.