Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial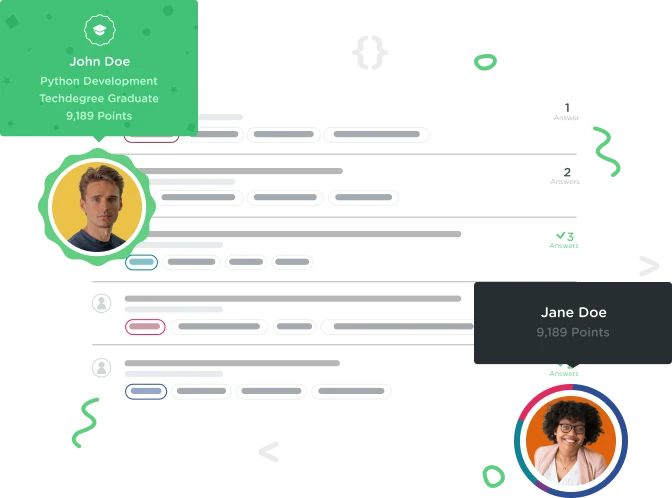

Pan Fu
Courses Plus Student 846 Pointswhat to use for unpacking dictionary and tuple?
i think i get the point but no idea about the quiz
2 Answers
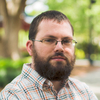
Kenneth Love
Treehouse Guest TeacherWe've used tuple and dictionary unpacking twice in the videos, both times for the .format()
method on a string. Do you remember what operators we used to achieve that?
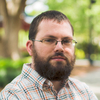
Kenneth Love
Treehouse Guest Teacherx, y, z = (1, 2, 3)
isn't really unpacking, it's multiple assignment. We're assigning multiple variables at once. Unpacking is turning one variable into multiple variables without creating those variables.
For example:
def add_nums(x, y):
return x + y
This function expects two variables, x
and y
. I can call it like add_nums(2, 4)
, of course. Or maybe I don't have 2
and 4
and I only have variables (a
and b
) I've captured from a user or another function. Then I could call it with add_nums(a, b)
.
Still not unpacking anything, though. But what if I just have a list of sets of numbers. Something like:
nums = [(1, 2), (5, 10), (82, 15), (70032, 8823)]
And I want to call add_nums
for each of those (I won't use map()
here since I haven't taught it yet, but feel free to look it up)?
output = []
for num_set in nums:
output.append(add_nums(*num_set))
Now output
will have the sum of each of those sets and I didn't have to use num_set[0]
and num_set[1]
. I unpacked the variables from the current version of num_set
into the function call.
So what about packing? Packing is actually a bit simpler because it's even more optional. Let's make another function.
def lt_gt(num):
return num - 1, num + 1
So lt_gt(5)
would give me back (4, 6)
(because commas make tuples, not parentheses). When I use this function, I can store the return value in two ways.
less, more = lt_gt(15)
Which will use multiple assignment to create less
and more
with the two values from the returned tuple. Or...
nums_around = lt_gt(15)
Which is make nums_around
equal to the tuple. This is considered packing because we've just put two explicit values into one variable.
You could, of course, then use these two together. add_nums(*lt_gt(15))
and get 30
.

Pan Fu
Courses Plus Student 846 PointsThank you again. So the point is:
1) multi-variable = single variable --- multiple assignment if explicitly
2) multi-variable = single variable --- unpacking if implicitly
3) single variable = multi-variable --- packing
for example:
a,b = (1,2) is multiple assignment because it assigns 1 and 2 to a and b by calling a=1,b=2.
So, if using multiple assignment in function add_nums(), it looks like:
a,b=(1,2)
add_nums(a,b)
alternatively,
add_nums(*num_set) is unpacking because it let the parameters a and b be the number in num_set directly without calling a = 1, b = 2 or a,b=1,2.
Am I right?
I would be very happy if you can answer more simple questions. I have downloaded the official Python 3.4.1 IDE(or not an IDE?), but it's very different from IDE like Eclipse. I think it's not very friendly. Could you give some information about it. I know that I can simply search from Google, but it would be perfect if you can provide a guideline. I am trying to figure out what will happen if unpacking a 4-length variable to 3 variables, but i got problems with compiling script in the official IDE, so please help.
And shall I take python 2 or python 3? I aim to use python for data analyzing and data mining. So which is better?
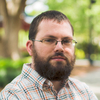
Kenneth Love
Treehouse Guest TeacherPan Fu Yeah, you have a good grasp on it. After awhile you don't really think about it, it just becomes a natural part of using Python.
By IDE, do you mean IDLE? It's really just a smarter text editor. Using something like emacs, vim, Sublime Text, or PyCharm will get you as much or more features as IDLE. If you're used to Eclipse, I'd suggest trying PyCharm.
We're only going to be teaching Python 3 here at Treehouse. I don't see the point in learning or teaching Python 2 any more since it has had an end-of-life announced (2020) and will only be getting security updates in the future. That said, there are still some data science libraries that are Python 2 only, so you might want to have both versions installed.
Pan Fu
Courses Plus Student 846 PointsPan Fu
Courses Plus Student 846 Pointsit's the * and **. Thank you so much! But could you explain more on packing and unpacking? cuz i tried to find answers from video Tuple Packing and Unpacking, and thought " x,y,z = (1,2,3) " is the unpacking. Could you give formal definitions for both of them?