Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial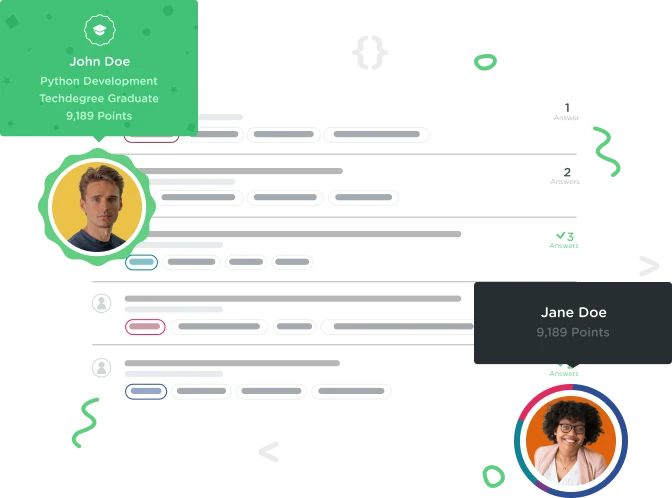
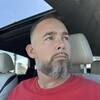
Justin Olson
13,792 PointsWhat's the difference?
During this video, we were shown how to create and call a random number function. In the video, the code was as follows:
function alertRandom() {
var randomNumber = Math.floor( Math.random() * 6 ) + 1;
alert(randomNumber);
}
alertRandom();
However, towards the beginning of the video, I paused it and decided to try this myself without instruction and came up with this:
function alertRandom() {
alert(randomNumber);
}
var randomNumber = Math.floor( Math.random() * 6 ) + 1;
alertRandom();
Mine has the var outside of the function, but it runs the same way. My question is...what is the real difference from using one technique instead of the other? Thank you! :)
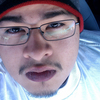
Chyno Deluxe
16,936 Points//Fixed Code Presentation
3 Answers

luis betancourt
1,236 PointsI would use the first example to write that function. When a variable is inside a function, it only exists within the scope of the function. The global scope stays clean. For small project you could do either the 1st or 2nd example but for larger projects the 1st example would be better.
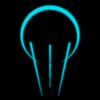
Jake Lundberg
13,965 PointsThe main difference here, is that in the first example (the code from the video) you are going to get a new random number every time the function is called. This is because the Math.random() function is called each time the function is executed. But in your code, the variable is going to hold the same random number no matter how many times the function is called because the Math.random() function is called only once...
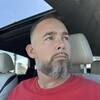
Justin Olson
13,792 PointsThank you everyone for the input. Definitely makes more sense now. This is why I love this site! :)
Joel Hernandez
Front End Web Development Techdegree Student 2,571 PointsJoel Hernandez
Front End Web Development Techdegree Student 2,571 PointsIn your coding the variable is a global variable & can be used again and again throughout the code. So to call a random number you would just use the randomNumber variable.
In the example, the variable is only ran inside of the function, for purpose of demonstrating a function, If you were to try to call the variable outside of the function, it wouldn't work. Example, if you were to try to do a document.write action and call (randomNumber), it wouldn't work because that variable is only ran INSIDE the function.
In reality, the way you did it would be the way most JavaScript programmers would use it. However, this example he used was a very basic function in JavaScript. As you continue the videos you will learn more about calling a variable inside functions vs setting them as global.