Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial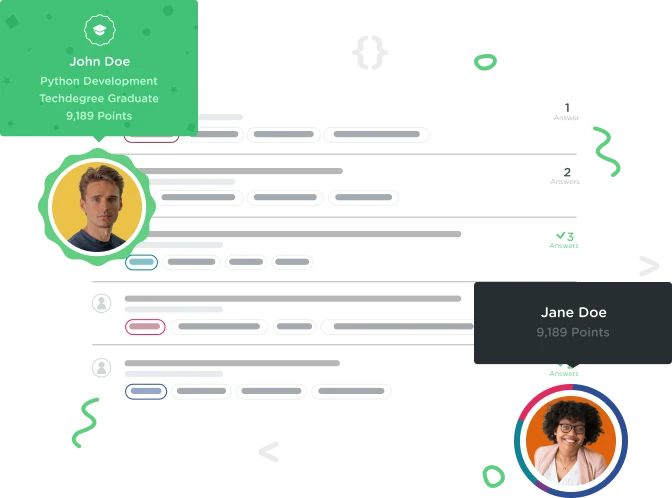

John Cuddihy
5,493 Pointswhats up with the "falsey"
Where are the faults coming through
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
while start is null
if even_odd(num) == 0:
print("{} is even!").format(num)
else:
print("{} is odd!").format(num)
start -=
7 Answers
Chris Grazioli
31,225 PointsI got this to work after playing with it a bit:
import random
start=5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
while start:
number=random.randint(1,99)
if even_odd(number)==1:
print("{} is even".format(number))
else:
print("{} is odd".format(number))
start-=1
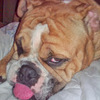
Shane Robinson
7,324 PointsI'm not entirely sure I understand what your question is, but there is an issue I see right away in your code:
start -=
should have something after the equal sign, i.e.
start -= 1

John Cuddihy
5,493 PointsThis is whats required: Alright, last step but it's a big one. Make a while loop that runs until start is falsey. Inside the loop, use random.randint(1, 99) to get a random number between 1 and 99. If that random number is even (use even_odd to find out), print "{} is even", putting the random number in the hole. Otherwise, print "{} is odd", again using the random number. Finally, decrement start by 1.
But everytime I hit check it says theres an error with task 1 which was just to import random, ive added the 1 to the -=, but no luck

Sahil Sharma
4,791 PointsWhat you probably meant to use as the statement for the while loop is while start is not None
notice the not as we want to end the loop as soon as start becomes 0, BUT what you need to understand is None and 0 are NOT the same thing in fact they are quite different, 0 represents an integer with value 0, but None keyword implies absence of any value inside a variable so using while start is not None
is actually wrong as we want to end the loop as soon as start reaches 0 not when start becomes 'empty'. Don't forget the colon after every block (you missed out after the while statement) and indent the line start -= 1 so that it is inside the while loop as we want start to decrement with every iteration of the loop and not after the loop is executed.

John Cuddihy
5,493 PointsAlright, last step but it's a big one. Make a while loop that runs until start is falsey. Inside the loop, use random.randint(1, 99) to get a random number between 1 and 99. If that random number is even (use even_odd to find out), print "{} is even", putting the random number in the hole. Otherwise, print "{} is odd", again using the random number. Finally, decrement start by 1. I know it's a lot, but I know you can do it!
Oops! It looks like Task 1 is no longer passing. Get Help Recheck work Go to task One even.py
1 import random 2 ā 3 start = 5 4 ā 5 def even_odd(num): 6 # If % 2 is 0, the number is even. 7 # Since 0 is falsey, we have to invert it with not. 8 return not num % 2 9 while start != 0: 10 num = random.randint(1,99) 11
12 if even_odd(num) == 0: 13 print("{} is even!").format(num) 14 else: 15 print("{} is odd!").format(num) 16 start -= 1
I've tried is not and != and it keeps referring to task 1 failing
Chris Grazioli
31,225 PointsHey John just a tip... the forum uses Markdown which has a formatting all its own, but the simple way to cut and display your code in formatted color is using this:
text for your comments or questions followed by a line break, then you just use three backticks followed by the language you want to be formatted (in this case python, but you could do html,etc), then your code starts on a new line, and follow it up with a newline containing 3 more back ticks
your copied code goes here
Chris Grazioli
31,225 PointsI keep getting the same error "task 1 failing" I cut and copy the code and burn my way back through the import that was there already then it passes. I do he same thing for the second task and it passes, but then I past back in the rest of my code and the interpreter starts spitting back errors about 'NoneType' object has no attribute 'format', or the fucntion which is clearly defined not being defined... I even tried moving the function def above the while loop but that doesnt work either
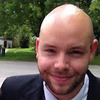
peterpyne
10,932 PointsIn the context of this challenge, could someone please explain how:
1)
while start:
is different than
2)
while start == True:
AND how
3)
while start != True:
is different than 1) and 2)?
I get that 1) is shorthand for saying "While Start is True", I don't get why 2) is False when start = 5, and I don't get why 3) runs 4 times through the While loop in this challenge. Can't quite tell what's going on.
Thanks!
Chris Grazioli
31,225 PointsIt probably has to do with the weirdness of True, truthy and 1!
Chris Grazioli
31,225 PointsPut another way, when you compare something to True or NOT True, its looking for strictly On or Off, 1 vs O. Its weird but when you use the shorthand its not as strict??? If you really want to go down the rabbit whole start playing around with negative numbers.... some of this stuff is enough to make you bleed from the nose
Chris Grazioli
31,225 PointsChris Grazioli
31,225 PointsFalsey is boolean False!
In boolean or anything computer related: True is also 1 or anything greater than zero really (this would be "Truthy") True is the same as 1 or even the same as ON which is why on power switches on certain appliances you sometimes will see what looks like a 1 and a 0.
False is 0 or off
So a short cut to writting the while loop is:
while start:
This will make a while loop that evaluates start, essentially asking the question "Is the condition after while true" Since start=5 to begin with and is therefor "truthy" you will notice that the code inside the while loop runs. It will continue to do so until the value of start becomes "falsey" ie: start=0 this is where your decrementing of the start variable at the end of the while loop will eventually cause the loop to end