Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial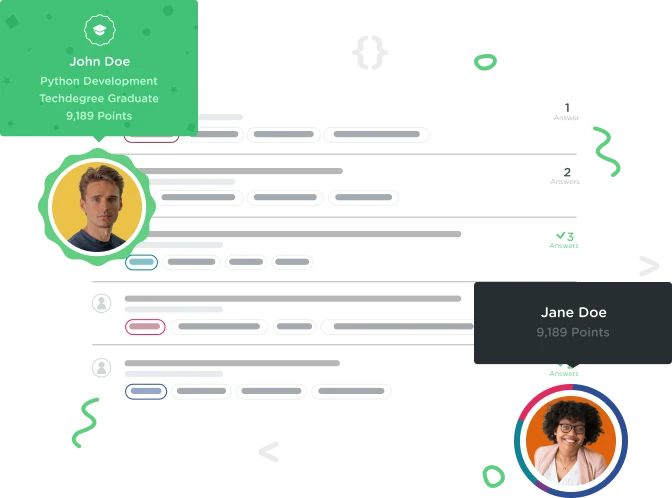

Arun Patel
1,180 PointsWhat's wrong with the letters being returned
What's wrong with the letters being returned
def disemvowel(word):
word = list(word)
word = [letter.lower() for letter in word]
try:
word.remove("a")
word.remove("e")
word.remove("i")
word.remove("o")
word.remove("u")
except ValueError:
pass
word = ", ".join(word)
return word
2 Answers
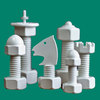
Steven Parker
231,236 PointsA few issues stand out at first glance:
- the entire word is converted to lower case, but the letters not removed are expected to stay as they were
- the list version of "remove" only removes the first item that matches
- when a match for any letter is not found, no remaining letters will be removed
- using ", " as the join string doesn't reconstruct the list into a word

Arun Patel
1,180 PointsThanks Steven. Thanks Ronaldo for the detailed explanation and clarification. This helped clarify my query.
Ronald Lira
12,217 PointsRonald Lira
12,217 PointsHey, a couple of comments.
It is a good practice (and a good idea) to use a different variable for doing any changes on your input variables unless you really know what you are doing, otherwise, you lose your original data.
word_lst = list(word)
Also, at this point, you already have a list. If you want to lower case your input, do it before splitting your data.
word_lst = list(word.lower())
However, note that this will lowercase all the letters, not just the vowels.
Then, you are trying to remove vowels in your
try
statement. However, the methodremove()
only remove the first occurrence of the argument from the calling array. In other words, if you have for example:As you can see only the first
a
was removed. How do you deal when you have the same vowel multiple times?For the previous example, your final output should be:
Note that the letters keep their original case.
My solution:
Usually, people solve this using a regular expression, but you are probably not familiar with that yet. Also, I see you are trying to use python list comprehensions, which are really powerful, so I will keep the same style:
Or which is the same:
Hope this helps,
Ronald