Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial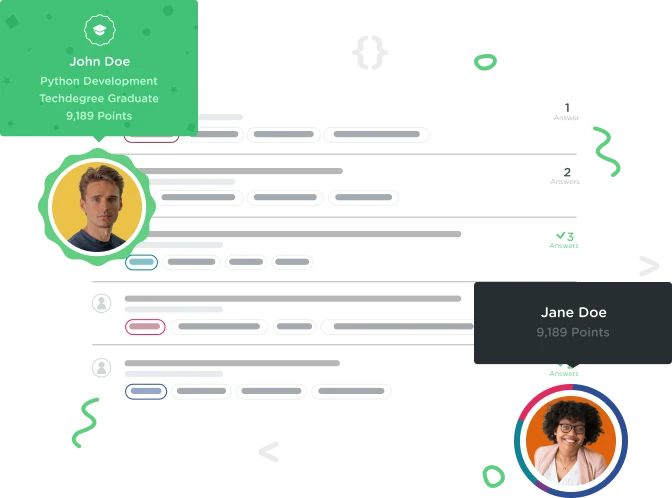
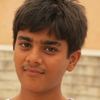
ashok bishnoi
10,160 Pointswhat's wrong with these codes?\
Use a for or while loop to iterate through the values in the temperatures array from the first item -- 100 -- to the last -- 10. Inside the loop, log the current array value to the console.
var temperatures = [100,90,99,80,70,65,30,10];
var i;
for (i = 0; i < tempratures.length; i+=1) {
console.log(tempratures);
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
6 Answers
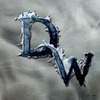
Hugo Paz
15,622 PointsHi ashok,
You have 2 typos.
The array name is temperatures, you have tempratures twice in your for loop.
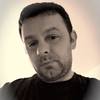
Jerko Begic
6,990 Pointstry this: var temperatures = [100,90,99,80,70,65,30,10]; for (var i = 0; i < temperatures.length; i++) { console.log( temperatures[i] ); }
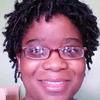
Martina Carrington
15,754 PointsJerko Begic thanks :D it work

James Kim
8,475 Pointswhen you console.log(temperature), you want to log the index as well... your "i" variable. So set up your for loop like this...
for(var i = 0; i<temperature.length; i++){
console.log(temperature[i]);
}
without the [i]... javascript is just going to print the last item in your array as it loops through. So with [i], it will print out all the items in the array one by one.
Hope that works outs.
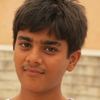
ashok bishnoi
10,160 Pointsvar temperatures = [100,90,99,80,70,65,30,10];
var i;
for (i = 0; i < temperatures.length; i+=1) {
console.log(i);
now...
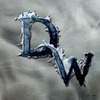
Hugo Paz
15,622 PointsYou need to show the temperature in your console log. [i] represents the index of the array temperature. How do you access a value in an array?
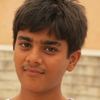
ashok bishnoi
10,160 Pointsvar temperatures = [100,90,99,80,70,65,30,10];
var i;
for (i = 0; i < temperatures.length; i+=1) {
console.log(temperatures);
}
now i am still wrong
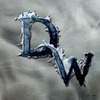
Hugo Paz
15,622 PointsYou need to loop through all the indexes in the array. temperatures[i]