Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial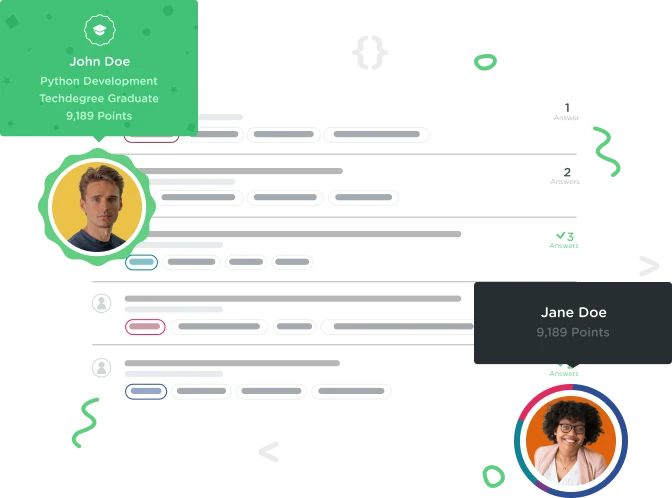
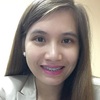
Agnes Caringal
6,239 Pointswhat's wrong with this code?...for or while
best technique to learn Javascript...
var temperatures = [100,90,99,80,70,65,30,10];
for (i var = 10, i < students.length, i = 100) {
console.log('temperature');
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
3 Answers

Greg Kaleka
39,021 PointsHi Agnes,
I definitely recommend taking another look at the video.
There are quite a few issues with your code:
- You haven't defined
students
, you've definedtemperatures
. - You've swapped the order of
var
andi
- Your for loop uses commas, but should use semicolons
- Take a look at the logic you have and think through it. Assuming you've changed it to
temperatures
, the first time through, you set i=10, then check to see if i is less than temperatures.length, which is 8. 10 is not less than 8, so it doesn't run. - You then set i=100, which happens after the first loop, so even if you had a longer array (such that i<temperatures.length), it would only run once. Actually, if you had an array with more than 100 elements, it would run forever, spitting out the 10th element once, then the 100th element an infinite number of times (not actually infinite - it would just crash your browser :)
- You are just logging the string "temperature", rather than logging any data.
What you're trying to do requires indexing. Indexing is a way to access specific elements in an array. We'll start with the first element, which is index 0, and work our way to the last one:
var temperatures = [100,90,99,80,70,65,30,10];
for (var i = 0; i < temperatures.length; i += 1) { // the i+=1 is how we step through the array 1 by 1
console.log(temperatures[i]); // this will log the item at index i each time through
}
Good luck!

Tristan Gaebler
6,204 PointsYou declared variable i like "i var" it should be "var i ="

Seth Kroger
56,413 PointsWhat your for loop does start at a value of 10; checks if 10 is less that the length of the array, and sets to value to 100 for all future runs through the loop. Depending on the length of the array the loop will either never run, run only once, or run forever.
The essence of a for loop is that the loop variable should change every time through the loop to a different value. Normally if you are looping over an array would start at i=0 (the first index) and add 1 every time through.
for( var i=0; i<array.length; i++) {
// do something with array[i]
}
(Also, the 3 statements in the for loop are separated by semi-colons, kinda like a regular JavaScript statement would.)
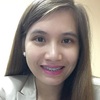
Agnes Caringal
6,239 Pointsthanks for the info. I will rewatch :)