Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial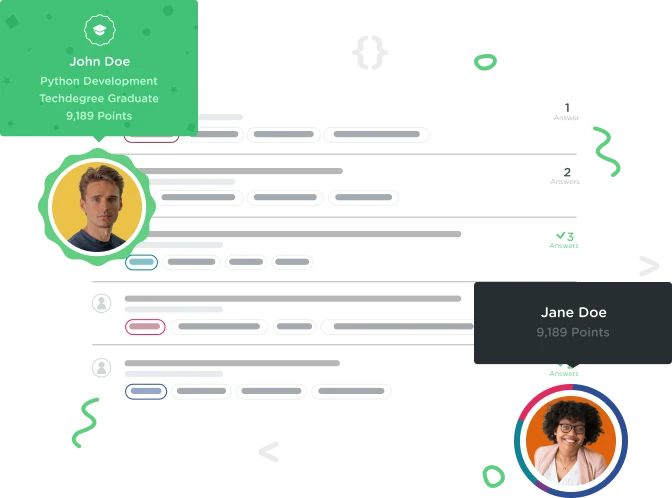

Vic Mercier
3,276 PointsWhen would I couldn't use a while loop instead of the do while loop?
I don't really understand.
1 Answer
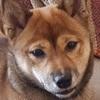
Katie Wood
19,141 PointsHi there,
Loops can be confusing at first - the short answer is that you generally CAN use a different type of loop for most situations, depending on how you structure your code.
The main thing to keep in mind is that certain loop types can be easier to code/easier to read in certain circumstances. Do-while loops can be useful when you know you want the code to run at least once (a while loop will not run at all if the condition it checks for is false beforehand, but a do-while loop will run once before it checks). A for loop is one of the easier ones to read and organize when you want a loop to repeat a particular number of times using a counting variable. You can do this with a while loop too, but a for loop can look cleaner and more organized. Finally, a while loop is best when you want the code to run only when certain conditions are met, and stop when they're not.
As you keep coding, you'll start to see circumstances that fit the different loop types. Sometimes you'll run into a scenario where it's really just up to your preference.
Hopefully this helped clear things up a little bit - happy coding!
Vic Mercier
3,276 PointsVic Mercier
3,276 PointsFollow this link to see what I mean:You could do the same thing as a do while using a while loop Link: https://teamtreehouse.com/community/in-the-example-mentionned-in-the-video-why-couldnt-we-do-that-instead-of-the-do-while-loop
Katie Wood
19,141 PointsKatie Wood
19,141 PointsFor anyone else reading this, this is the code from that link:
You could use a while loop for this, but the code above has a problem - it doesn't prompt for the password again. You just say "Please try again", which doesn't let the user enter a new password. The above is an infinite loop - if the user enters the incorrect value the first time, they will just get an endless stream of alerts that tell them to try again, unless they refresh the page to start over. The user's response also shouldn't be a const value - if they enter it wrong, you want them to be able to try again, which would change the value. A while loop that does prompt the user again would look like this:
This would work. Notice, though, that we now have two very similar lines of code. Therefore, a do while loop does make sense:
In this example, the "Please try again" message will just appear if a wrong password has been entered - if they enter nothing or if it's the first prompt, it won't appear. This code repeats itself less because there's only one line that prompts for the password. The two are very similar though - this is a situation where you could use the while loop if you really wanted to - the do-while is just a bit "DRYer".
Now, though, we can actually mimic what we've accomplished with the do-while loop by just initializing the variable at the top of the while loop's code, without the prompt:
This does effectively the exact same thing as the do-while, and it doesn't repeat itself either. The do-while loop just makes some logical sense because a password prompt is always something you're going to want to do at least once, which is pretty much a textbook example for when you use a do-while. Ultimately, though, it's up to you as the programmer which one more easily expresses what you're trying to do - there are almost always multiple ways to do what you want to do.
Does that make sense?
Vic Mercier
3,276 PointsVic Mercier
3,276 PointsThank you for your other answer!
Vic Mercier
3,276 PointsVic Mercier
3,276 PointsWhy is that important:Now, though, we can actually mimic what we've accomplished with the do-while loop by just initializing the variable at the top of the while loop's code, without the prompt:
Katie Wood
19,141 PointsKatie Wood
19,141 PointsIf you mean why pointing that out is important, it was just intended as an example, since you asked about while loops specifically - I wanted to make it clear that in this case you could do it with a while loop and be fine.
If you mean why initializing the variable outside the loop without the prompt is important, it was mostly to avoid repeating code. It doesn't matter much in a small example like this, but on a larger program, repeating code can get messy. It doesn't need to prompt for the value up there, because it will happen down in the loop. I still initialized it outside the while loop for two reasons:
There are multiple ways to do almost anything, so what it usually comes down to is what makes the code the most self-documenting - that means that you can tell what the code is intended to do by looking at it, minimizing the need for lengthy comments.
This example is small, so having an extra line of code or a repetition isn't going to matter, but having code that is neat and organized (non-repetitive and as easy to read as possible) is an awesome habit to get into, because it will make your lengthier programs much easier to maintain down the line.