Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial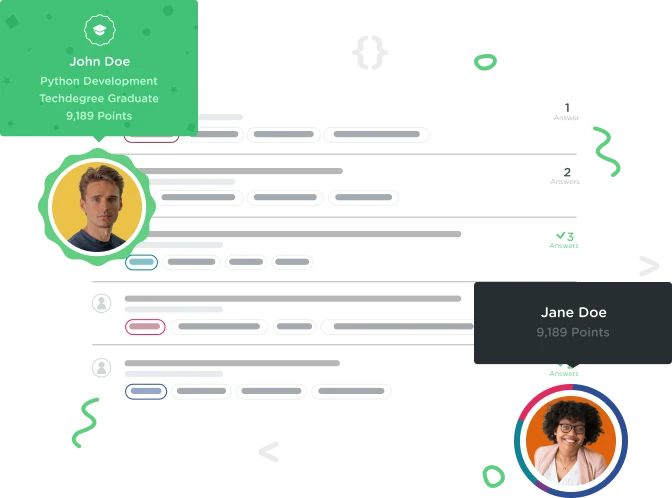
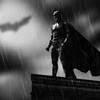
William Barrows
915 PointsWhere did I go wrong???
I am pretty sure I have the logic right here, just not the correct formatting. I am confused as to where curly braces are suppose to go with conditional statements. Thanks ahead of time for any help understanding this!
<?php
$studentOneName = 'Dave';
$studentOneGPA = 3.8;
$studentTwoName = 'Treasure';
$studentTwoGPA = 4.0;
//Place your code below this comment
if ($studentOneGPA < 4.0);
echo $studentOneName " has a GPA of" $studentOneGPA;
elseif ($studentOneGPA == 4.0);
echo $studentOneName " made honor roll";
if ($studentTwoGPA < 4.0);
echo $studentTwoName " has a GPA of" $studentTwoGPA;
elseif ($studentTwoGPA == 4.0);
echo $studentTwoName " made honor roll";
?>
2 Answers

Yousif Sayer
7,073 PointsThe issue is you are not using the concatenation between variables ans string.
You have to use (.)dot between variable and string , similar to this echo $studentOneName . " has a GPA of" . $studentOneGPA;
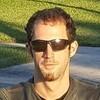
Joshua Kaufman
19,193 Points<?php
$studentOneName = 'Dave';
$studentOneGPA = 3.8;
$studentTwoName = 'Treasure';
$studentTwoGPA = 4.0;
//Place your code below this comment
if ($studentOneGPA == 4.0)
{echo $studentOneName . " made the Honor Roll";}
else{
echo $studentOneName . " has a GPA of " . $studentOneGPA;}
if ($studentTwoGPA == 4.0){
echo $studentTwoName . " made the Honor Roll";}
else{
echo $studentTwoName . " has a GPA of " . $studentTwoGPA;}
?>
I'll do my best to break down all your errors. First, and probably most importantly ALL IF, ELSEIF, and ELSE statements must contain opening and closing curly brackets. You'll notice I inserted 4 pairs of curly brackets because there are 4 conditional statements in my code. In addition, you should see 6 dots in my code which allows the strings and variables to be written together in an echo statement in PHP. Inside the double quotes, you should notice that there is a spacebar press after the first quote and before the second. This is to ensure that this happens:
Treasure made the Honor Roll and NOT Treasuremade he Honor Roll or EVEN Davehas a gpa of3.8
Lastly, make sure you are writing it as the prompt requests for your string parts of your echo statements. Treehouse code challenges are VERY particular, so make sure you are writing it as they request.
Hope this helps!
Yousif Sayer
7,073 PointsYousif Sayer
7,073 PointsThe issue is you are not using the concatenation between variables ans string.
You have to use (.)dot between variable and string , similar to this echo $studentOneName . " has a GPA of" . $studentOneGPA;