Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial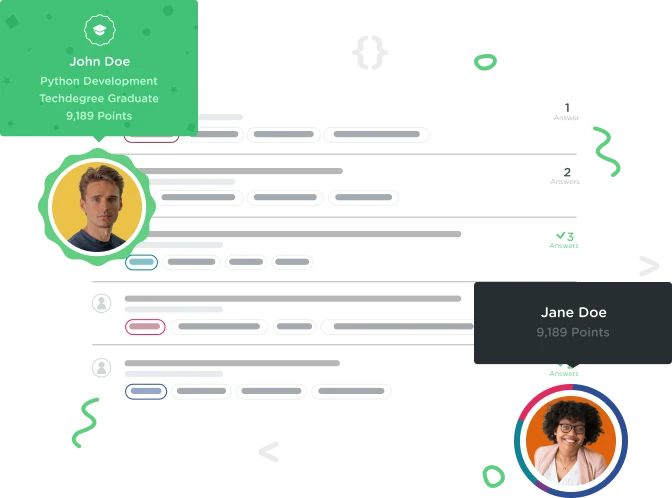
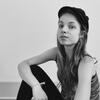
Kate Johnson
Python Development Techdegree Graduate 20,155 PointsWhile loop not working.
Hello, I am writing a function that takes user input and then sets a password according to their preferences. However, the "while" loop is not working when I am trying to set the password length. The function works when setting the characters, but there seems to be an issue with setting the length. It always falls into the "default" option, no matter what you pass into the prompt. I tried changing while (length === undefined) to while (length === 0) and that did not solve the problem either. Any help would be greatly appreciated, please! Thank you!
var password = "";
// characters object
var characters = {
lowercase: "abcdefghijklmnopqrstuvwxyz",
uppercase: "ABCDEFGHIJKLMNOPQRSTUVWXYZ",
numeric: "0123456789",
special: "!+'@,-./:;<=>?@[]^_{}~#$%^&*()"
};
// Function to set password characters and length
var setPasswordCharacters = function() {
// when prompt answered input validated and character type selected
var alphabet, numbers, special;
var length = 0;
while(length === undefined) {
var promptLength = window.prompt("Please enter your password length between 8 and 128 characters.");
promptLength = parseInt(promptLength);
switch (promptLength) {
case (8 <= promptLength && promptLength <= 128):
length = promptLength
break;
default:
window.alert("You need to provide a valid answer. Please try again.");
break;
}
}
while (alphabet === undefined) {
var promptCase = window.prompt("Would you like your password to include UPPER case letters? Enter 'YES' or 'NO.'");
switch (promptCase.toLowerCase()) {
case "yes":
alphabet = characters.lowercase + characters.uppercase;
break;
case "no":
alphabet = characters.lowercase;
break;
default:
window.alert("You need to provide a valid answer. Please try again.");
break;
}
}
while (numbers === undefined) {
var promptNumeric = window.prompt("Would you like your password to include numbers? Enter 'YES' or 'NO.'");
switch (promptNumeric.toLowerCase()) {
case "yes":
numbers = characters.numeric
break;
case "no":
numbers = ""
break;
default:
window.alert("You need to provide a valid answer. Please try again.");
break;
}
}
while (special === undefined) {
var promptSpecial = window.prompt("Would you like your password to include special characters? Enter 'YES' or 'NO.'");
switch (promptSpecial.toLowerCase()) {
case "yes":
special = characters.special
break;
case "no":
special = ""
break;
default:
window.alert("You need to provide a valid answer. Please try again.");
break;
}
}
// set password characters based on prompt responses
password = alphabet + numbers + special;
return;
};
// Function to shuffle password characters and set length
var shuffle = function() {
var passwordArray = [];
// convert password to an array
var passwordArray = password.split("");
// randomly sort array items
passwordArray = passwordArray.sort(() => Math.random() - 0.5);
// set password length from setPasswordLength()
passwordArray.length = length
// convert passwordArray back to string
password = passwordArray.join("");
return;
}
// FUNCTION TO GENERATE PASSWORD
var generatePassword = function() {
// prompt and ask for password inputs
setPasswordCharacters();
// shuffle characters and set length
shuffle();
// password displayed in an alert
window.alert("Your new password is " + password);
};
// Get references to the #generate element
var generateBtn = document.querySelector("#generate");
// Write password to the #password input
function writePassword() {
generatePassword();
var passwordText = document.querySelector("#password");
passwordText.value = password;
}
// Add event listener to generate button
generateBtn.addEventListener("click", writePassword);
2 Answers
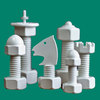
Steven Parker
231,008 PointsSince length
was initially set to 0, it's never undefined
. But testing for 0 should work.
But you cannot have a statement like "case (8 <= promptLength && promptLength <= 128):
". A "case" only takes a literal value. For testing with expressions like this, use if
instead of switch
.
So, is this your homework?
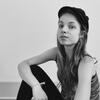
Kate Johnson
Python Development Techdegree Graduate 20,155 PointsLove a recursive function :D thank you for the help
Kate Johnson
Python Development Techdegree Graduate 20,155 PointsKate Johnson
Python Development Techdegree Graduate 20,155 PointsYes, this is my homework! Thank you so much. Do you know how I can make the if statement recall the prompt for length if someone enters a number that is not between 8 and 128? Thak you so much!
Kate Johnson
Python Development Techdegree Graduate 20,155 PointsKate Johnson
Python Development Techdegree Graduate 20,155 PointsActually I figured it out!
Steven Parker
231,008 PointsSteven Parker
231,008 PointsGood job!