Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial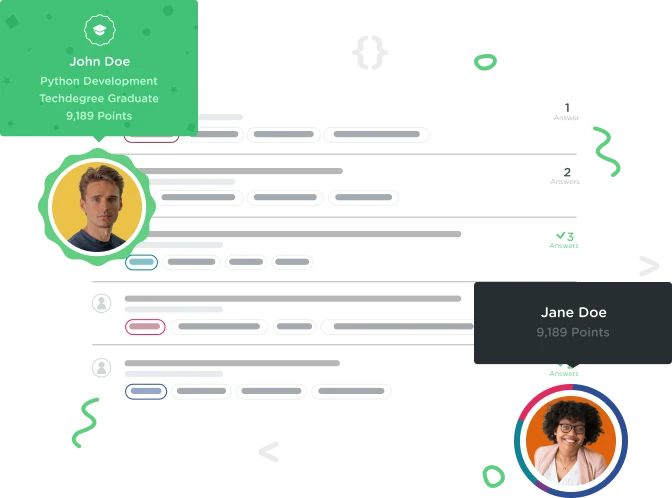

Andrei Oprescu
9,547 PointsWhy am I getting an error?
I have this code which is just the same as Kenneth's:
import random
def game():
secret_num = random.randint(1, 10)
guesses = []
while len(guesses) < 5:
try:
guess = int(input("Guess a number between 1 and 10: "))
except ValueError:
print("{} isn't a number".format(guess))
else:
if guess == secret_num:
print("You got it! My number was {}".format(secret_num))
break
elif guess > secret_num:
print("Too high!")
elif guess < secret_num:
print("Too low!")
guesses.append(guess)
else:
print("You didn't get it! My number was {}".format(secret_num))
play_again = input("Do you want to play again? Y/n ")
if play_again != 'n':
game()
else:
print("Bye!")
game()
And I was testing this code, I stumbled across an error:
Guess a number between 1 and 10: a
File "number_game.py", line 8, in game
guess = int(input("Guess a number between 1 and 10: "))
ValueError: invalid literal for int() with base 10: 'a'
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "number_game.py", line 30, in <module>
game()
File "number_game.py", line 10, in game
print("{} isn't a number".format(guess))
UnboundLocalError: local variable 'guess' referenced before assignment
As you can see, I tried to see if the except 'ValueError' worked. And it didn't. Can you help me understand why this is happening?
Thank you
1 Answer
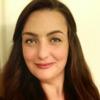
Jennifer Nordell
Treehouse TeacherHi there! This bug is intentional. Check out the Teacher's Notes where Kenneth asks if you spotted the bug. Kudos on that by the way. Not everyone spots it.
Here's what's happening: inside your try block you prompt the user for a number and then try to turn it into an integer and assign the result to the variable guess
. But if that fails then the assignment to guess also fails.
In the except
block you then try to print a string to the user formatting it with the value stored in guess
, but because the assignment failed, guess
is undefined.
There are a couple of ways to fix this.
You could simply change the error message to something like:
print("That was not a number!")
Or if you're set on displaying what the user input you're going to need to put the prompt assigning the value to guess outside the try
block and only include the conversion to an integer in the try
. Take a look:
while len(guesses) < 5:
guess = input("Guess a number between 1 and 10: ") # assign whatever they type to guess
try:
guess = int(guess) # try and convert their input to an integer
except ValueError:
print("{} isn't a number".format(guess)) #if the value cannot be converted to an integer print out what they typed
Hope this helps!
Andrei Oprescu
9,547 PointsAndrei Oprescu
9,547 PointsThank you very much! It helped a lot!